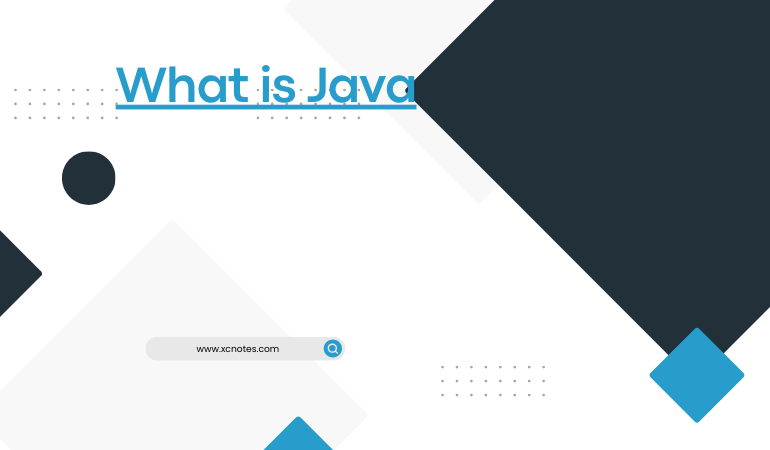
What is Java
- Java is a 3rd generation(3GL first appeared in 1950s) programming language(consist of series of English words) which implements the concepts of OOPs. It inherits most(but differs from both) of the features of the exciting language C and C++. In addition, it also adds new features to make it one of the simple and easy-to-learn object-oriented languages. One of the main feature of Java is that it is a platform independent language, i.e.a program written for one system can be executed on any other system (WORA-Write Once Run Anywhere(run on multiple Operating Systems) and a popular language for developing Easy Web/Internet applications like java applets.
- Easy Web/Internet applications like java applets.
JAVA programming language was developed by Sun Microsystems(an American technology company) in 1991(developed by the team headed by James Gosling),. This language was originally called, “Green talk”, After that, it was called “Oak”but was renamed(OAK was already a trademark) “Java” in 1995.
JAVA is a computer language to specify textual representation by writing source code, which obeys the rules of the Java programming language. The source code is saved in a text file with an extension (.java). Therefore, C and C++ developers should migrate to Java easily. But Java programming language also has its difference from C and C++ concepts that need to be taken care of when migrating to Java e.g Java doesn’t support Operator Overloading, Pointer etc.
What is Java used for and so Popular?
Some important Java applications are:
- It is official programming language for developing Android Apps(Android games,3-Dimensional games, cannot built without Java)
- Helps you to create Enterprise Software like banking applications.(J2EE)
- Wide range of Mobile java Applications(J2ME(Micro Edition-created for mobile phones, tablets, etc)
- Distributed Applications(software that runs on multiple(more than one) computers within a network like JINI (Java Intelligent Networking Infrastructure) gives an infrastructure implements a mechanism called JavaSpaces)
- Use for Big Data Technology(Scala (Scalable Language), a pure object-oriented language, is based on Java.)Python, used in Big Data. An open framework with Big Data is called HADOOP(written in Java)
- Java Programming of Hardware devices(TV, Remote)
- Used for Server-Side Technologies(Back-end (Web Applications)) like Apache, Hibernate, etc.
History of Java Programming Language
Here are the main landmarks from the history of Java:
- The Java language was initially called OAK.
- Originally, it was developed for handling portable devices and set-top boxes. Oak was a massive failure.
- In 1995, Sun changed the name to “Java” and modified the language to take advantage of the burgeoning www (World Wide Web) development business.
- Later, in 2009, Oracle Corporation acquired Sun Microsystems and took ownership of three key Sun software assets: Java, MySQL, and Solaris.
Java Versions
Here is a brief history of all the Java versions with their release date.
Java Versions(Evolutions) | Release Date |
JDK Alpha and Beta | 1995 |
JDK 1.0(java development kit) | 23rd Jan 1996 |
JDK 1.1 | 19th Feb 1997 |
J2SE 1.2(java 2standard edition) | 8th Dec 1998 |
J2SE 1.3 | 8th May 2000 |
J2SE 1.4 | 6th Feb 2002 |
J2SE 5.0 | 30th Sep 2004 |
Java SE 6 | 11th Dec 2006 |
Java SE 7 | 28th July 2011 |
Java SE 8 | 18th Mar 2014 |
Java SE 9 | 21st Sep 2017 |
Java SE 10 | 20th Mar 2018 |
JAVA SE 11 | 25th Sep 2018 |
JAVA SE 12 | 19th Mar 2019 |
JAVA SE 13 | 17th Sep 2019 |
JAVA SE 14 | 17th Mar 2020 |
JAVA SE 15 | 15th Sep 2020 (latest Java Version) |
Java Editions…..
- J2SE-to develop standalone applications or applets(Standard edition)
- J2ME-to develop an application for mobile devices(Mobile edition).
- J2EE=to develop server-side applications like java servlets & JSP(Enterprise edition).
- JavaFX-to develop rich internet(lightweight) applications
What is Java Programming
Java Programming(Java Program Structure)
A java program may contain following sections :
- Documentation section : //Messge print(Comments-single line)
/*….tells about program….*/(Comments-Multi lines)
- Package section :
A statement that declares the package name and informs the compiler that the classes defined here belong to this package.
package pack ;
- Import statements (import java.awt.*);
This statement instructs the interpreter to load the class contained in the package.
import student .class-name ;
- Interface statements :
It is like a class that includes a group of method declarations.
- Class Definitions : (class message)
Classes are the essential elements of the Java program
The use of multiple classes is defined in this section.
- Main method class
{
Main Method Definition
}
Every java stand-alone program requires a main method(public static void main(String aa[]) as its starting point. The main method(String aa[]) creates objects of various classes and establishes communication between them.
First Program in JAVA
/*…A simple program….*/
class first
{
public static void main (String aa[])
{
System.out.println (“Xcnotes.com”);
}
}
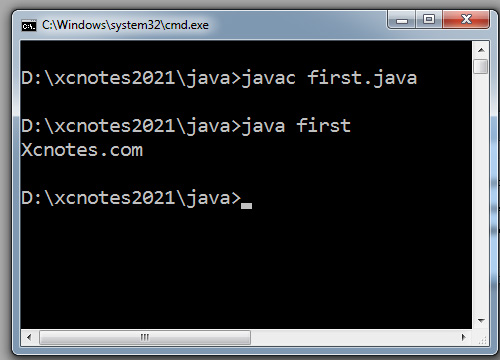
How to Compile and Run Java program
- To compile the program, we must use javac, with the name of the source file on the command line as :
javac first .java
after removing the errors, the compiler creates a file called first .class containing the byte codes of the program.
- Java interpreter is used to run a stand-alone program as :
java first
Features(Characteristics) of Java
- Simple
No use of Pointer, Operator Overloading, header files,
- Object-Oriented
Include basic concepts-Object, class, Inheritance, Abstraction, polymorphism, Encapsulation. Object & class contain the program code and data.
- Distributed
Java makes us suitable to retrieve files by calling the methods from any machine on the internet. Java can handle TCT/IP protocols
- Platform Independent
Executed on any machine(Mac/Os, Windows, Linux), can run on different OS(operating system) and CPU.
- Interpreted
Java compiler generates byte-codes, that are translated onto the machine-readable instructions in runtime(JVM)
- Robust
Provide automatic garbage collection, exception handling mechanism to handle the exception,
- Portable
U can carry the java code to any platform like a window to Linux or other.
- Multithreaded
U can write Java programs that deal with many tasks at once by defining multiple threads(many tasks simultaneously.)
- Secure
It doesn’t allow a programmer to create a pointer.
…………An Example of Object Oriented is……….
class rectangle //name of the class is a rectangle
{
int l,b; // integer variable l and b
public void getdata( int x, int y) //function with arguments x and y(formal argument)
{
l=x; //value passes from x to l
b=y; //value given to b
}
int rectarea() //function without argument
{
int area=l*b; //calculation to find the area of rectangle
return(area); //return is used because of int function rectarea()
}}
class rect //main class
{
public static void main(String aa[])
{
int area; //integer variable area is used to call the function rectarea because the function is return type
rectangle r11=new rectangle(); //r1 is the object of class rectangle
r11. getdata (2,12); /*calling function getdata and value give to formal argument this is the
actual arguments*/
area=r11.rectarea(); //calling function rectarea the output is given to variable area
System. out. println (“area=”+area); //print output
}}
Save as……..rect.java
Run ……..javac rect.java
………java rect
Output…….area=24(l*b (l=2,b=12))
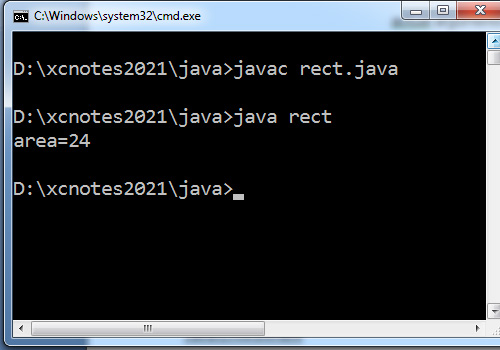
Java Environment
- Java includes many development tools(JDK), classes, and methods(JSL-Java standard library) also known as API(Application Programming Interface)
- JDK-tools like Java Compiler, Interpreter, etc.
- API-Includes classes & methods grouped into many packages like awt package, lang package, io package.