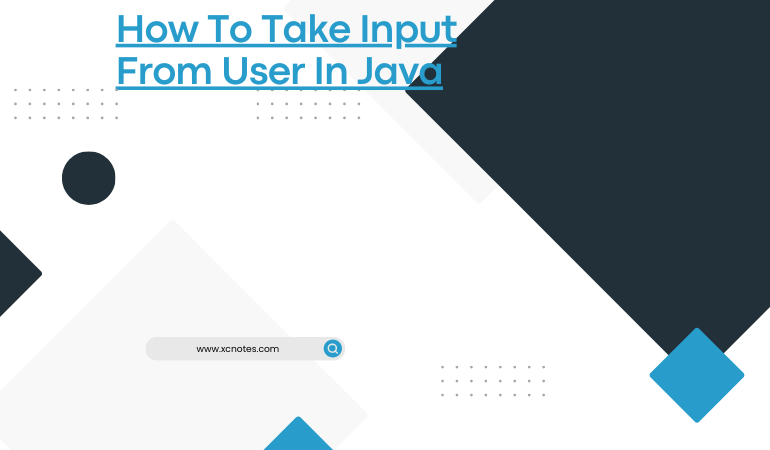
How To Take Input From User In Java
In this tutorial, I am going to show you how to take input from users in java using two different ways. You can follow any method (Scanner or BufferedReader) according to your convenience. To understand how we get input from users in java we first must know of the following two things :
System. out – Java uses System. out to refer to the standard output device.
System.in – Java uses System. in to refer to the standard input device.
By default output device is monitor and the input device is the keyboard. To perform console output, we simply use println(System.out.println()) method to display a primitive value like int, float, double, or a string(group of characters like “name”) to the console. Console input is not directly supported in Java, but we can use the Scanner class to create an object to read input from System.in or we can use BufferedReader from the java.io package.
Taking Input From User In Java Using Scanner Class
Taking Input Using Scanner Class : Scanner input = new Scanner( System.in )
The above line: Scanner input = new Scanner( System. in ) creates a scanner object and assigns its reference to the variable input.
Note: Don’t worry if you find it hard to understand what these things mean and what they are doing. Once we come to the topic classes and objects then you’ll understand everything.
Various methods can be invoked to take various types of input.
Method | Description |
nextByte( ) | reads an integer of type byte |
nextShort( ) | reads an integer of type short |
nextInt( ) | reads an integer of type int |
nextLong( ) | reads an integer of type long |
nextFloat( ) | reads a number of type float |
nextDouble( ) | reads a number of double type |
next( ) | reads a string that ends with a white space character |
nextLine( ) | reads a line of text ( ends when Enter key is pressed ) |
Now let’s do a simple program that takes the input of type int, double, and string. All other input can be taken in the same manner.
// Taking input of type int, double, and string
import java.io.*;
public class TakeInput
{
public static void main(String[] args)throws IOException
{
int number;
double decNumber;
String text;
BufferedReader input=new
BufferedReader(new InputStreamReader(System.in));
System.out.print(“Enter an integer number : “);
number = Integer.parseInt(input.readLine());
System.out.print(“Enter a decimal number : “);
decNumber = Double.parseDouble(input.readLine());
System.out.print(“Enter a line of text : “);
text = input.readLine();
System.out.println(“Ingeger Number : ” + number);
System.out.println(“Decimal Number : ” + decNumber);
System.out.println(“Text : ” + text);
}
}
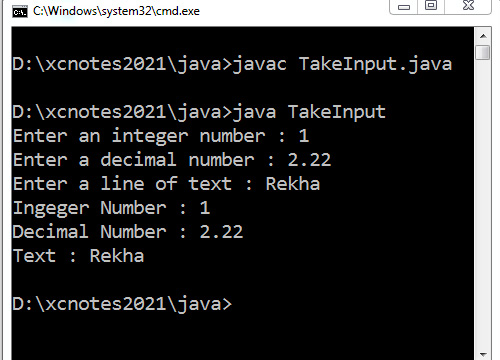
Now in the very first line, I have imported the Scanner class which is in java. util package. ( import java.util.Scanner )
Taking Input From User In Java Using BufferedReader Class
Now let me show you another method to take input from the user.
BufferedReader input = new BufferedReader( new InputStreamReader ( System.in ));
// Taking input of type int, double and string
//String Input in Scanner Class
import java.util.Scanner;
public class TakeInputScanner
{
public static void main(String[] args)
{
int number;
double decNumber;
String text;
Scanner input = new Scanner(System.in);
System.out.print(“Enter an integer number : “);
number = input.nextInt();
System.out.print(“Enter a decimal number : “);
decNumber = input.nextDouble();
System.out.print(“Enter a string like name: “);
text = input.next();
System.out.println(“Integer Number : ” + number);
System.out.println(“Decimal Number : ” + decNumber);
System.out.println(“Text : ” + text);
}
}
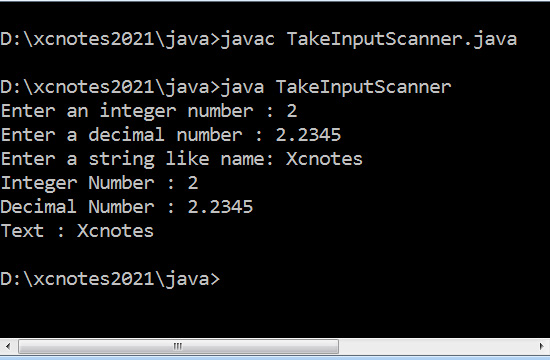
The code import java.io.*; imports all classes from java.io package. In the above programs I have used System.out.print( ) and System.out.println( ).
System.out.println( ) – prints the text in it and moves the cursor to the next line.
System.out.print( ) – prints the text in it but does not move the cursor to the next line.