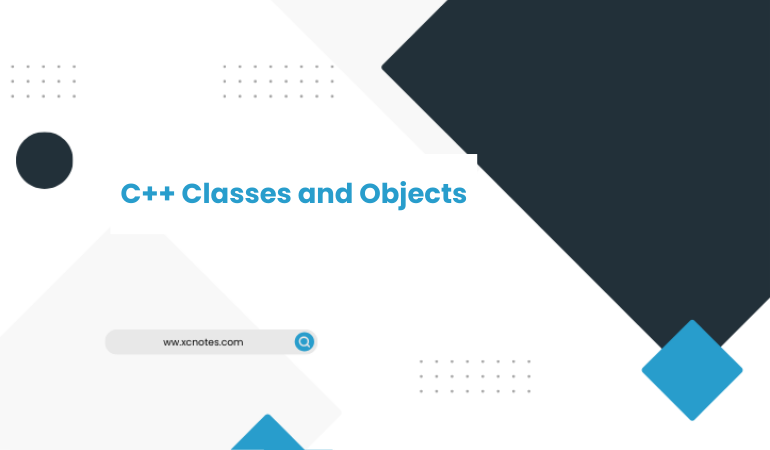
C++ Classes and Objects
In C++, classes and objects are fundamental concepts that support object-oriented programming (OOP). Here’s a brief overview of classes and objects in C++:
Classes:
In C++, a class is a user-defined data type that allows you to encapsulate data members and member functions into a single unit. Classes are the building blocks of object-oriented programming (OOP) and provide a way to model real-world entities and their behavior.
A class is a user-defined data type in C++ that represents a blueprint for creating objects. It encapsulates data members (variables) and member functions (methods) that operate on those data members. The general syntax for a class definition is as follows:
class ClassName {
public:
// Data members (attributes)
dataType member1;
dataType member2;
// Member functions (methods)
returnType method1(parameters);
returnType method2(parameters);
// …
};
public: Access specifier that defines the visibility of the members. Members declared as “public” (access specifier)can be accessed from outside the class.
Data Members:
Data members are variables declared within the class. They represent the attributes or properties of the class. Data members can have different data types.
Member Functions:
Member functions are functions declared within the class. They define the behavior or actions associated with the class. Member functions can manipulate the data members and perform various operations.
Objects:
An object(abc ob1(ob1 is the object of class abc)) is an instance of a class, created based on the class blueprint. Objects represent real-world entities and can have their own unique data and behavior. To create an object, you use the class name followed by an object name and optional parentheses:
Here’s the syntax:
ClassName objectName; // Object creation
Example:
Here’s a simple example of a C++ class and object:
#include <iostream.h>
#include<conio.h>
// Define the class
class Circle {
public:
// Data members
double radius;
// Member function to calculate area
double calculateArea() {
return 3.14 * radius * radius;
}
};
void main() {
// Create an object of the Circle class
Circle myCircle;
// Set the radius of the circle
myCircle.radius = 5.0;
// Calculate and display the area
cout << “Area of the circle: ” << myCircle.calculateArea() << endl;
getch();
}
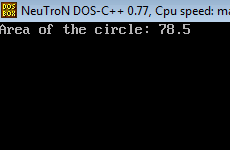
In this example, the Circle class has a data member radius and a member function calculateArea(). An object myCircle is created, and its radius is set to 5.0. The area of the circle is then calculated and displayed.