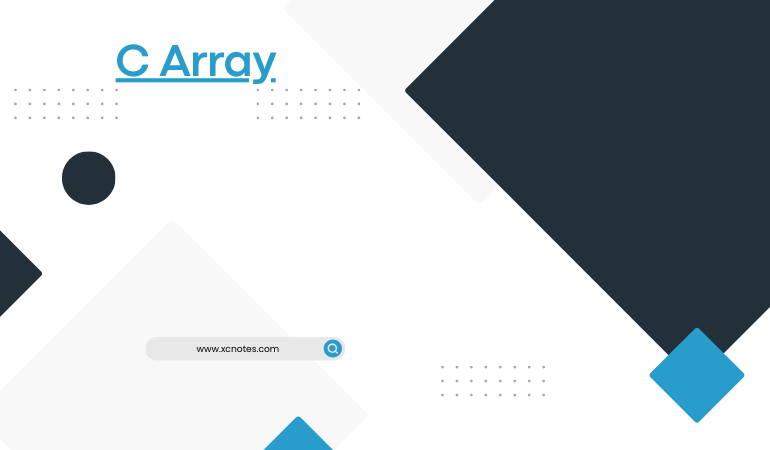
C Array
What is an Array?
An array is also known as a list because it is a list of values that are indexed by a number. The data of the array is stored in a linear list that is addressed differently by the index given in the square brackets.
C Array: Declaration & Initialization
We can initialize the elements in the array(int as[]) in the same way as the ordinary variables(like int as) when they are declared.
Initialization of C Array:
Type array_namee[size]={list of valuees};
The values in the list {2,5,6}are separated by commas, here the values are 2 5 and 6
for example :
Int numb[3]={0,0,0};
Will declare the array size(numb[3]) as a array of size 3 and will assign zero(0) to each element if the number of values in the list is less than(<3 starts with 0) the number of elements([0],[1],[2]), then only that many elements are initialized(numb[3]).
In the declaration of an array the size may be omitted, in such cases the compiler allocated enough space for all initialized elements For Example, the statement
Int coounter[]={1,1,1,1};
Will declare the arrays to contain 4 elements with initial values 1. This approach works fine as long as we initialize every element in the array.
Examples of Arrays:
- int *myCArray = new int[3];//dynamic
- int myCArray[3];//static
- myCArray[0] = 87;
- myCArray[1] = 94;
- myCArray[2] = 92;
- if(myCArray[0]>myCArray[1])
- {
- }
- int Sum = myCArray[0]+myCArray[1]+myCArray[2]
Notice that the name of the array is used followed by brackets and an index, each index is a different variable. To assign myCArray[0] to 87 does not affect the value at myCArray[1].
The initialization of arrays in C suffers from 2 drawbacks:
- There is no appropriate (suitable)way to initialize only selected elements.
2. There is no shortcut(time-saving method) method to initialize a large number of elements.
How to Use an Array
To use the array, the variable name is followed by square brackets with an int index in the middle. For example, theList[2]; refers to the third element in the array. The third is because the indexing starts at 0. It is very common to use an int, short, or char variable to address the array. For example, theList[i] where i is an int with a value within the range of the array.
C Program to count Positive(+ve) and Negative(-ve) in an Array
#include<stdio.h>
#include<conio.h>
void main()
{
int a[50],nn,count_neg=0,count_pos=0,i;
clrscr();
printf(“enter the size of the array”);
scanf(“%d”,&nn);
printf(“enter the elements of an array”);
for(i=0;i<nn;i++)
scanf(“%d”,&a[i]);
for(i=0;i<nn;i++)
{
if(a[i]<0)
count_neg++;
else
count_pos++;
}
printf(“these are %d negative(-ve) nos in the array\n”,count_neg);
printf(“these are %d positive(+ve) nos in the array\n”,count_pos);
getch();
}
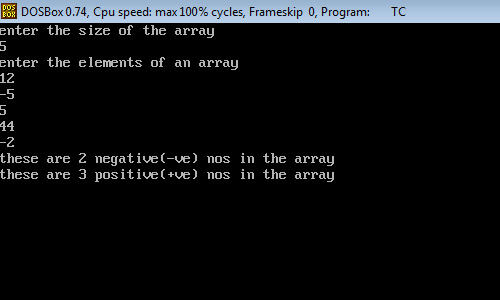
Algorithm of the above program
Step 1: The main() method is start.
Step 2: Initialize an array a[] and variable I, nn;
Declare count_neg and count_pos. //for a negative and positive number and assign with value 0
Step 3: Input the size of an array nn. for example 5
Then input the element of an array. //through a loop and continue till 0 to n.
Example 2 4 -5 9 -2
Step 4: Loop start till 0 to nn. //loop for the count of the positive and negative array.
Now check the condition: if(a[i]<0) increment the count_neg by 1. Else increment the count_pos by 1.
Step 5: Now print the count of negative and positive in an array.
Example 2 4 9
-5 -2
Overview: Types of Arrays
Static Arrays: These are a fixed size with no need for memory management. Arrays like these are quick and easy to use because there is no C memory management to deal with. Examples of static arrays:
- int myCArray[3];
- myCArray[0] = 7;
- myCArray[1] = 8;
- myCArray[2] = 9;
- int myCArray2[5] = {6,7,8,9,10};
Dynamic Array: These arrays are created dynamically to the size specified and need to be deleted. A dynamic array is addressed just like a static array but does require C memory management.
- int *myCArray = new int[3];
- myCArray[0] = 7;
- myCArray[1] = 8;
- myCArray[2] = 9;
- delete[]myCArray;//always delete when done
2d Array in C
Multidimensional Array: This type of array can be either static or dynamic. Basically, Multidimensional Arrays are arrays of arrays. The notation is very similar, just add on an additional set of brackets and index. See the following examples to help make sense of all this.
Static Example:
- int myCArray[3][3];
- myCArray[0][0] = 7;
- myCArray[0][1] = 8;
- myCArray[0][2] = 9;
- myCArray[1][0] = 17;
- myCArray[1][1] = 18;
- myCArray[1][2] = 19;
- myCArray[2][0] = 27;
- myCArray[2][1] = 28;
- myCArray[2][2] = 29;
- int myCArray2[2][2] = {{6,7},{8,9}};
Dynamic Example:
int **dynArray = NULL;
dynArray = new int*[3];//Pay close attention to this
if( dynArray == NULL ) {return false;}
for(int i=0; i<3; i++)
{
dynArray[i] = new int[3];
for(int ik=0; ik<3; ik++)
{
dynArray[i][ik] = 0;
}
}
Array Program in C(Example)
This example of a static C array of int that checks for the lowest score, drops it, and averages the rest.
#include<stdio.h>
#include<conio.h>
void main()
{
int scores[5];
int average = 0;
int lowest = 10000;
int i;
clrscr();
scores[0]=88;
scores[1]=90;
scores[2]=92;
scores[3]=85;
scores[4]=98;
for(i=0;i<5;i++)
{
if( scores[i] < lowest )
{
lowest = scores[i];
}
average += scores[i];
}
average -= lowest;
average /= (5-1);
printf(“lowest=%d”,lowest);
printf(“\nAverage=%d”,average);
getch();
}
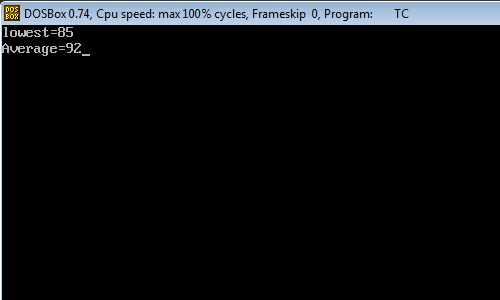
Фурнитура для газели
Wow, amazing blog layout! How long have you been blogging for? you make blogging look easy. The overall look of your site is great, as well as the content!
кофейная заварка
Hi friends, nice piece of writing and nice arguments commented at this place, I am really enjoying by these.
вот такая
Эта статья действительно заслуживает высоких похвал! Она содержит информацию, которую я долго искал, и дает полное представление о рассматриваемой теме. Благодарю автора за его тщательную работу и отличное качество материала!
Rekha Setia
thanku so much
пишут
I really like your blog.. very nice colors & theme. Did you make this website yourself or did you hire someone to do it for you? Plz answer back as I’m looking to design my own blog and would like to know where u got this from. thanks a lot
Rekha Setia
I designed this website myself