Before we talk about data type first we know about variables because data type is used with variables. Without variables the meaning of the data type is nothing, it’s incomplete. Data types are used when you create variables in the program. Each variable is assigned a data type.
Variable is the name that is used to reserve space in memory For example if we use
int a;
Then, ‘a’ is the variable of integer type. So, this is clear in the above example that without variable data type is not complete and without data type variable can do nothing. Data types define the type of data that the variable is an integer type, float type, character type, or so on.
Data types are of two types:
a) Primitive data type b) Non –primitive data type
Primitive data type—
The primitive data type is a predefined data type and its name is a reserved keyword.
When we talk about the data type then first we should know about the size of the datatype(how many bits(0,1) it can store, means its capacity or range).
Data Types in Java with Example
Java Primitive Data types are:
1)int-> size of integer data type is 32 bit(4 byte)
2)char-> size of character data type is 8 bit(1 byte).
3)float-> size of float(.value like 2.3)data type is 32 bit(4 byte).
4)byte-> size of data type is 8 bits(1 byte).
5)short- the size of the short data type is 16-bit (2 bytes).
6)long-> size of the long data type is 64 bits(4 bytes).
7)double-> size of double data type is 64 bits(4 byte).
8)Boolean-> it may return true or false its size is (1 bit)
There can be various types of data that can be used in our daily life to solve any types of problems, e.g………………….
- Integer values like 6,-56,-6789 etc.(decimal point & comma is not allowed)
- Floating values like 2.45,-5.789 etc;(which have a decimal point(.) in them)
Character
- like ‘c’,’ y’,’u’;( store the special character (ASCII)and alphabet)
- String(group of characters) like “good”,” many”;
- Array(Collection of same data types of data elements that have been given a common name)
To deal with all types of problems, different data types are available in the language.
Primitive Data Type Example
class datatype
{
public static void main(String aa[])
{
int a;
float b;
char c;
double d;
String e;
a=5;
b=12.45f;
c=’x’;
d=56.5677;
e=”xcnotes”;
System.out.println(“integer=”+a);
System.out.println(“float=”+b);
System.out.println(“character=”+c);
System.out.println(“double=”+d);
System.out.println(“string=”+e);
}
}
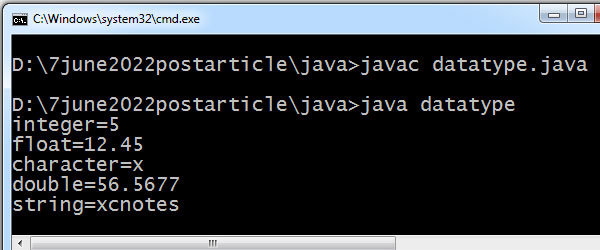
The Non Primitive data types are:
1)Classes
2)Interfaces
3)Array
Non-primitive data types like Array, Interfaces, etc are not predefined in any computer language like C, C++, or Java. It is also known as “Reference type”( or object reference).
The non-primitive data type is created by the programmer.
Non-Primitive Data Type example (Array example:)
int a[];//declaration of an array
………..Program example in an array…………..
class arr
{
public static void main(String aa[])
{
int i,le;//variable declaration ‘I’ for loop and le for count the length
int a[]={1,4,6,7,8,2};//constant value
le=a.length;
for(i=0;i<le;i++)
{
System.out.print(a[i]);
}
}
}
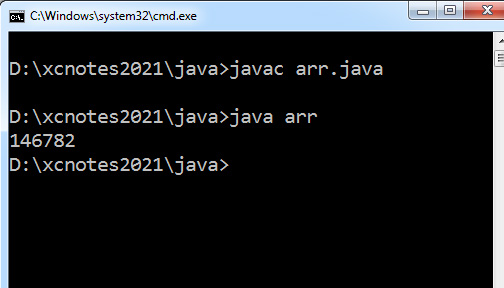
Primitive Data Type Example in Java
class primitive-data-type
{
public static void main(String aa[])
{
int sum;
float money;
char letter;
double a;
sum=30;
money=5.21f;
letter=’R’;
a=2.01E6;
System.out.println(“sum=”+sum);
System.out.println(“money=”+money);
System.out.println(“letter=”+letter);
System.out.println(“a=”+a);
}
}
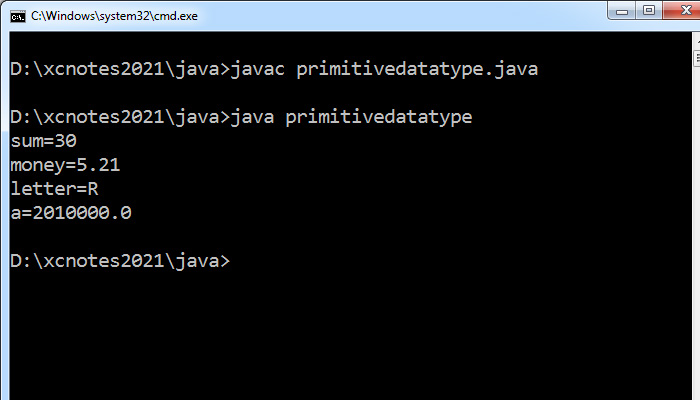