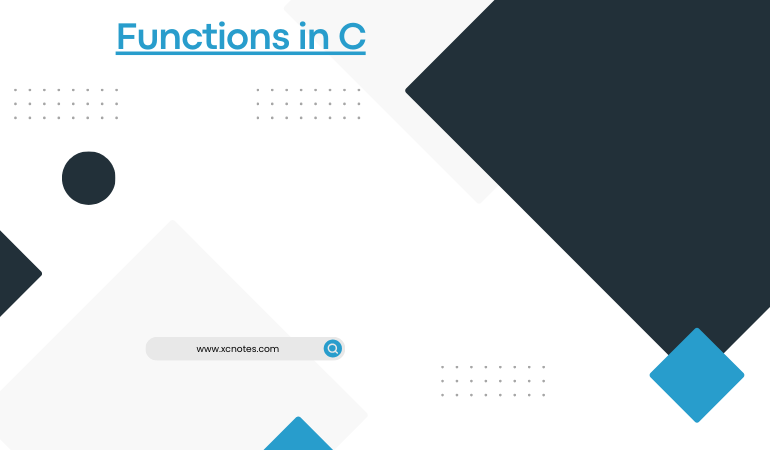
Functions in C
The need for C functions
When we write programs, sometimes it happens that we have to type any code repeatedly, so to avoid this situation, we create C functions. Creating function reduces the consumption of memory space and we can use that code in the program when needed. With the help of the function, we divide a large program into smaller parts, which also facilitates debugging the program.
What are C functions?
C functions are a group of many codes/instructions and statements that work together to execute a task.
Types of Functions in C(Categorization of C function)
1. Library function or pre-defined function
2. User-defined function
1. Library function in C
The library function is a pre-defined function in the C library. Library function includes header files like stdio.h, conio.h, string.h, graphics.h, dos.h, math.h etc. All header files we can include in our program using “#include ” and these header files include C functions.
2. User-defined function in C
These functions are made by themselves. You can also modify these functions. We will further understand how to create user-defined functions, but before that how do we create functions.
Function declaration
A function declaration(void f1()) is a process in which it tells the compiler about the function name, it’s return type and parameter list(int f2(int a,int b)). It is also called function prototyping.
Syntax
return type name_of_function (parameter list);
return type- return type tells the compiler the value you want to return is of which data type (int, float, double, char).
Function name- Function name is an identifier that is decided by the programmer.
Parameter list- This list declares the data type and arguments at the time of calling the function.
If we write declarations before the main function, then this prototype is called a global prototype and this declaration is available for all the functions written in the program.
When we write the declaration in the function definition then the prototype is called the local prototype.
Function definition
A function definition is a group of statements that are written in the function body.
Syntax
return type name_of_function (parameter_list)
{
Function body;
}
Calling function
When we call a function, the control goes to the function body.
Syntax
Function_name (argument_list);
C program which demonstrates the function.
#include <stdio.h>
#include<conio.h>
int add_function(int a, int b);
int main ()
{
int c;
clrscr();
//Calling of addition function
c = add_function(10,20);
printf (“c=%d”, c);
return 0;
}
//Function definition
int add_function(int a, int b)
{
int addition;
addition = a+b;
return addition;
}
Output
c=30
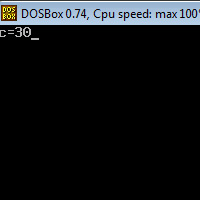
Call By Value & Call By Reference in C
Call by value
##include<stdio.h>
#include<conio.h>
//Declaration of maximum function
int maximun(int a, int b);
int main ()
{
int a = 11, b=21,c;
clrscr();
//Calling maximum function
c = maximum(a,b);
printf (“c=%d\n”,c);
return 0;
}
//Maximum function definition
int maximum(int a, int b)
{
int temp;
if (a>b)
{
temp=a;
}
else
{
temp=b;
}
return temp;
}
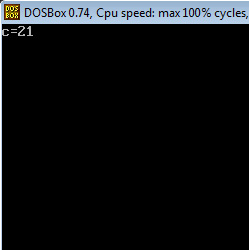
Explanation
In this function, we declare the value of the variable ‘a’ and variable ‘b’ in the main function, these values are stored in the stack memory. If we want to redeclare these values in the local function (maximum), no changes in the values of the local function. values of the variable in the main function will not change.
Output
c = 21
Call by reference
#include<stdio.h>
#include<conio.h>
// Declaration of function of swapping two numbers
int swap (int *a, int *b);
int main()
{
int x = 19, y=56;
clrscr();
// Calling function swap
swap(&x,&y);
printf (“x=%d\n y=%d\n”, x,y);
return 0;
}
//Function Definition of swap
int swap(int *a, int *b)
{
int temp;
temp = *a;
*a = *b;
*b = temp;
}
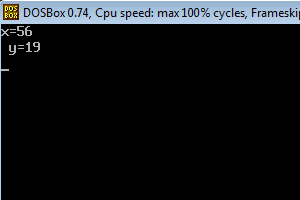
Explanation
In call-by-reference, the address of variables is assigned to the function as a parameter. We can change the value of the variables in the local function (swap). The same memory is used for variables declared in the main function or if you want to redeclare variables in the local function.
Output
x = 56
y = 19