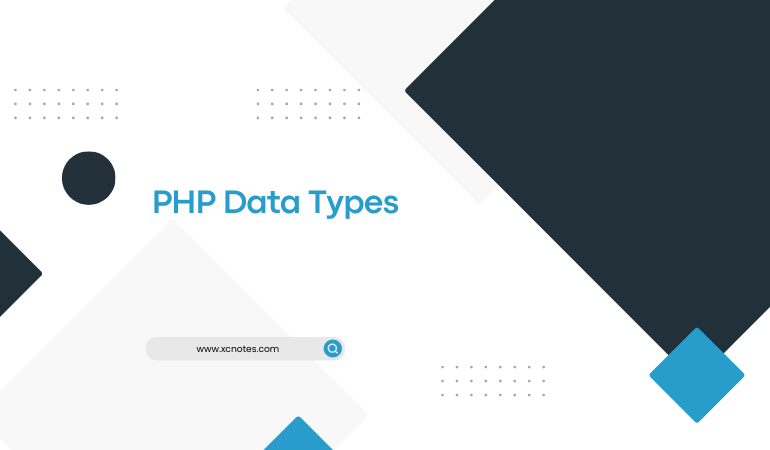
PHP Data Types
PHP DATA TYPES
In programming, if we want to use values(like 10,20) then we have to use data type(int, float, char) to represent its base type as real, integer, character, or Boolean that determines the possible values for that type. We can perform an action on values of that type. The meaning of data and the way values of that can be stored.
PHP supports eight primitive data types.
Four primitive types
- Boolean(true and false)
- Integer(3,5)
- Float(3.56,6.78)
- String(“xcnotes”)
Two compound types
- Array
- Object
finally two special types
- resource
- NULL
VARIABLE
A Variable is a temporary storage area for storing value given by the user or program. variable’s type depends on data types followed by programming language. In PHP $ sign represent a variable to store value and work with it.
Example:
<?php
$a=100;
echo $a;
?>
In PHP using any variable, we don’t need to the declaration of it(use $a,$b), because in PHP variables get the data type when we assign any value. In the above example, the integer variable is automatically defined after getting the same type value.
Naming conventions for PHP variables
There are the following rules for naming a PHP variable:
- All Variables in PHP start with a $ sign, followed by the name of the variable($a).
- A variable name must start with a letter or the underscore character “_”.
- A variable name can not start with a number(like $1a).
- A variable name in PHP can only contain alpha-numeric characters and underscores (A-Z, 0-9).
- A variable name can not contain spaces(like $first number).
Note: $ sign must be the first character in declaring the name of a variable using PHP.
Note: Variables in PHP are case sensitive so be careful when using $x or $X both are not the same name of a variable.
Float(decimal values like 45.67)
<?php
$many = 2.2888800;
$many_2 = 2.2111200;
$few = $many + $many_2;
print( $few);
echo “<br>”;
echo $few;
?>
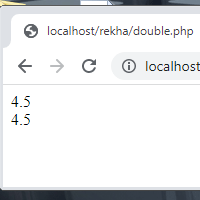
Boolean(true or false)
<?php
$true_value = true;
$false_value = false;
Print (“true_value = ” . $true_value);
Print (” false_value = ” . $false_value);
?>
String(sequence of character like”xcnotes”)
<?php
$variable = “xcnotes”;
$literally = “My $variable will not print!”;
print($literally);
$literally = “My $variable will print!\n”;
print($literally);
?>
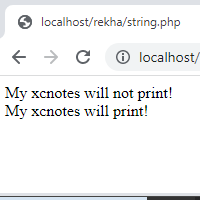
Two variables
<?php
$first_number = 10;
$direct_text = ‘Xcnotes ‘;
print($direct_text . $first_number);
?>
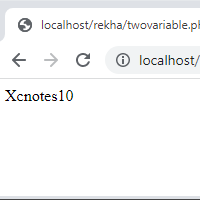
Array(Store multiple values in one variable)
<?php
$array = array(“name”=>”Toyota”,”type”=>”Celica”,”colour”=>”black”,”manufactured”=>”1991″);
$array2 = array(“Toyota”,”Celica”,”black”,”1991″);
$array3 = array(“name”=>”Toyota”,”Celica”,”colour”=>”black”,”1991″);
print_r($array);
echo'<br>’;
print_r($array2);
echo'<br>’;
print_r($array3);
?>
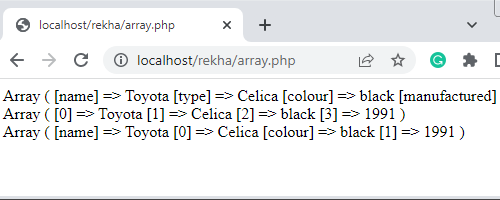