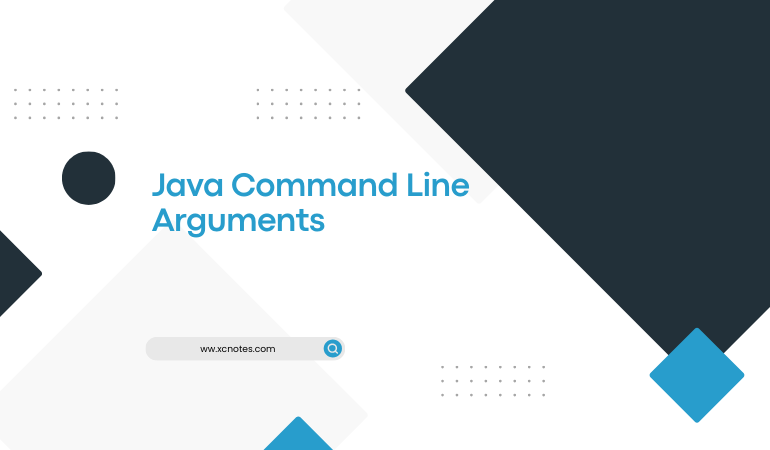
Java Command Line Arguments
In Java, command-line arguments can be passed to a program when executing it from the command line. These arguments allow you to provide inputs or configuration parameters to your Java program. Here’s how you can access command-line arguments in Java:
Command-line arguments are passed to the main method of your Java program as an array of strings.
The main method is like:
public static void main(String[] args) {
// Your code here
}
The argss
parameter((String[] args)) is an array of strings that represents the command line arguments.
The args parameter in the main method represents the array of command-line arguments. You can access individual arguments using array indexing (args[0], args[1], etc.).
To run a Java program with command-line arguments, you need to execute the program from the command line and provide the arguments after the program name. For example:
java YourProgram arg1 arg2 arg3
Here’s a simple example that demonstrates how to access and use command-line arguments in Java:
public class CommandLineExample {
public static void main(String[] args) {
// Check if arguments are provided
if (args.length > 0) {
// Access and print individual arguments
for (int i = 0; i < args.length; i++) {
System.out.println(“Argument ” + i + “: ” + args[i]);
}
} else {
System.out.println(“No arguments provided.”);
}
}
}
If you compile the above code into a file named CommandLineExample.java, you can execute it with command-line arguments like this:
java CommandLineArgumentsExample a1 a2 a3
In this example, a1
, a2
, and a3
are the command line arguments that will be passed to the program.
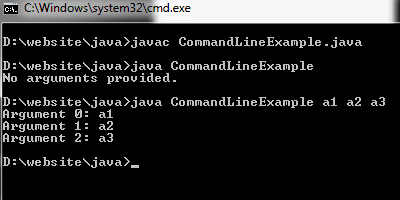
Example with output:
class command
{
public static void main(String aa[])
{
int cc, ii=0;
String stri;
cc=aa.length;
System.out.println(“Number of Arguments=”+cc);
while(ii<cc)
{
stri=aa[ii];
ii=ii+1;
System.out.println(ii+”:”+”Java is”+stri+”!”);
}
}
}
Explanation…
Here, aa is declared as an array of strings (known as string objects). Any arguments provided in the command line(at the time of execution)are passed to the array aa as its elements. To run this program…
Javac command.java
Java command basic, java
This command line contains four arguments. These are assigned to the array aa as follows…
Basic……..aa[0]
Java……..aa[1]
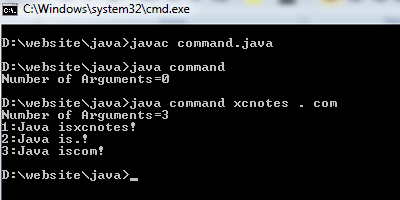