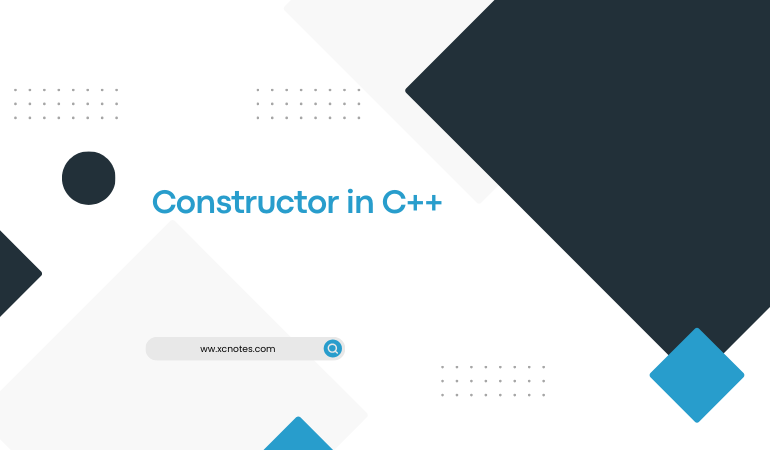
Constructors in C++
In C++, a constructor is a special member function of a class that is used to initialize the objects of that class. It is called automatically when an object is created, and its purpose is to set the initial values of the object’s data members or perform any necessary setup operations.
Here is the syntax for defining a Constructor in C++:
class MyClass {
public:
// Constructor declaration
MyClass() {
// Constructor body
// Initialize data members or perform setup operations
}
};
In the example above, MyClass
is the name of the class, and the constructor has the same name as the class itself. The constructor does not have a return type, not even void
.
Constructors can also have parameters to accept arguments during object creation. Such constructors are called parameterized constructors. Here’s an example:
class MyClass {
public:
// Parameterized constructor declaration
MyClass(int value) {
// Constructor body
// Initialize data members or perform setup operations using the parameter
}
};
In the example above, the parameterized constructor MyClass
takes an integer parameter named value
. This allows you to provide an initial value during object creation.
Constructors are useful for ensuring that objects are properly initialized and in a valid state when created. They can also be overloaded, meaning you can have multiple constructors with different sets of parameters, providing flexibility in object creation.
It’s worth noting that if a class does not explicitly define any constructors, C++ automatically generates a default constructor, which initializes the data members to their default values (e.g., zero for numeric types) and performs no additional operations.
Example with output….
#include<iostream.h>
#include<conio.h>
class abc
{
public:
abc() //constructor means class name and function name always the same
{
cout<<“good”;
}};
void main()
{
clrscr();
abc ob1;
getch();
}
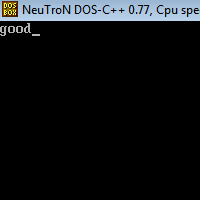
The above class has a member function with the same name as its class. Whenever an object of the “abc” type will be created(declared), the compiler will automatically call the constructor. Constructors have no return type, not even void.
#include<iostream.h>
#include<conio.h>
class rectangle
{
private:
int length,breadth,area;
public:
rectangle()
{
length=breadth=0;
}
rectangle(int a)
{
length=breadth=a;
}
rectangle(int a,int b)
{
length = a;
breadth = b;
}
void disp()
{
area=length*breadth;
cout<<“area=”<<area;
}};
void main()
{
rectangle r(0);
rectangle r1(5);
rectangle r2(2,5);
clrscr();
r.disp();
r1.disp();
r2.disp();
getch();
}
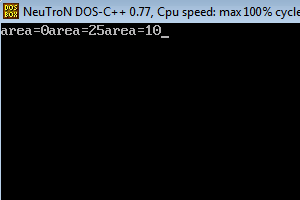