Loop is a sequence of instructions continuously repeated depending on the boolean condition. If we want to execute our statement repeatedly, we can implement loop techniques and put statements execution according to the boolean condition.
PHP Loop Types
While Loop
For Loop
Do While
PHP While Loop
While loop is the most accessible loop to implement re-execution statements continuously while the pre-specified condition is true. Loop depends on three major steps one initialization of the counter and second condition and the last one increment or decrement counter for testing condition.
Syntax of While Loop
while (condition)
{
Statements;
Increment or Decrement;
}
Example of While Loop
<?php
// Example of while loop by xcnotes.com
// repeat continuously until counter becomes 10
// output: ‘xxxxxxxxx’
$counter = 1;
while ($counter < 10)
{
echo ‘x’;
$counter++;
}
?>
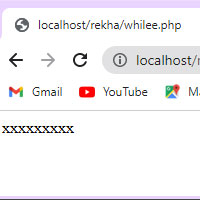
PHP For Loop
For loop used to re-execute coding block on specified steps that we are aware of it. It executes the coding block on specified steps.
PHP For Loop Syntax
for ( Counter Initialization; Condition ; Increment/decrement )
{
Statements;
Increment or Decrement;
}
PHP For Loop Example
<?php
// Example by xcnotes.com
// Table Printing of given no to $num variable
// output: ‘2 4 6 8 10 12 14 16 ‘
$num = 2;
for ($counter=1;$counter<=10;$counter++)
{
$table=$counter*$num;
echo “$table <br/>”;
}
?>
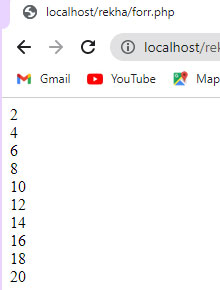
PHP Do While Loop
Do while loop is so different from for or while loop. It executes statements at least once, and then repeatedly executes the code block or not depending on the boolean condition.
PHP Do While Loop Syntax
do
{
Statements;
Increment or Decrement;
}
while(condition);
PHP Do While Loop Example
<?php
// Example by xcnotes.com
// repeat continuously until counter becomes 10
// output: ‘xxxxxxxxx’
$counter = 1;
do
{
echo ‘x’;
$counter++;
}
while ($counter < 10);
?>
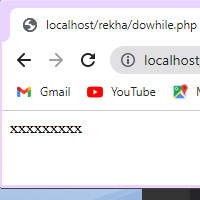