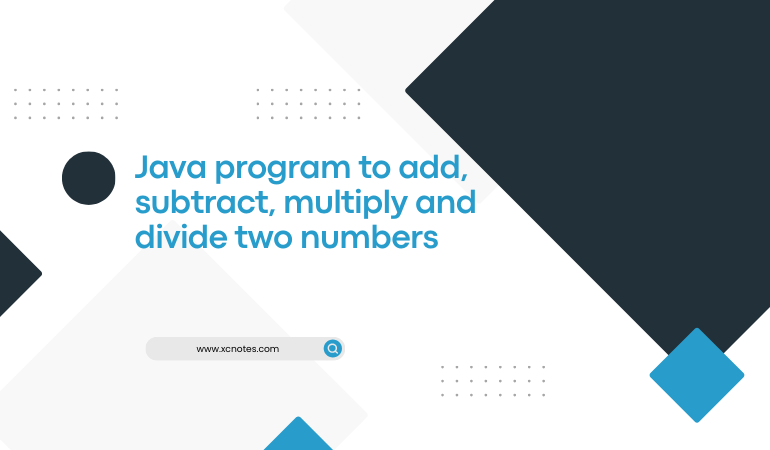
Java program to add, subtract, multiply and divide two numbers
The switch statement is a multi-way decision-making statement in which we can select only one . The switch statement starts with the “switch” keyword like (switch(expression)). Switch defines one or more case values which we know as case labels. Case labels consist of case keywords followed by a colon(:), and every case is terminated by a break statement.
Example 1. Java Program for Addition, subtraction, multiplying, and dividing two numbers using switch(Scanner class)
import java.util.Scanner;
class menu
{
public static void main(String asa[])
{
int aa, bb,choicee;
//create Scanner(Scanner class) object to object input from keyboard(Run Time Value)
Scanner inputt=new Scanner(System.in);
System.out.print(“Enter 2 (aa,bb)nums……”);
aa=inputt.nextInt(); //read the first number(aa)
bb=inputt.nextInt(); //read the second number(bb)
System.out.println(“1. ADDITION”);
System.out.println(“2. SUBTRACTION”);
System.out.println(“3. MULTIPLICATION”);
System.out.println(“4. DIVISON”);
System.out.println(“Pls enter the no. btw 1 to 4 for calculation(arithmetic +,-,*,%,/)”);
choicee=inputt.nextInt();
switch(choicee)
{
case 1:
System.out.println(“Sum ===”+(aa+bb));
break;
case 2:
System.out.println(“Sub ===”+(aa-bb));
break;
case 3:
System.out.println(“product ===”+(aa*bb));
break;
case 4:
System.out.println(“Div===”+(aa/bb));
break;
default:
System.out.println(“Enter number btw 1 to 4”);
}
}
}
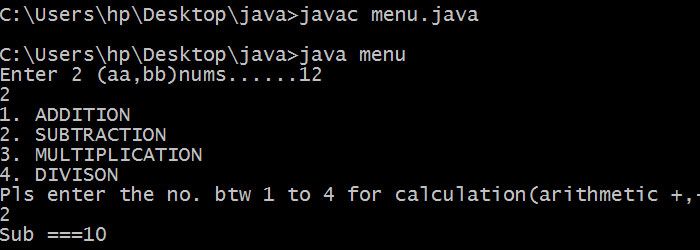
Explanation….
In this example, the user is prompted to input two numbers(aa and bb) and the values of choice whose value determines the type of the operation. The value of the choice entered by the user is compared with each case in the order they appear in the switch statement. When a match occurs, the statement to that case is executed and the break statement causes the immediate exit from the switch statement. If the value of the choice doesn’t match then the default case is executed.
Example 2. Java Program for sum, subtract, multiply and divide two numbers using switch(Command Line Arguments)
class arithmetic
{
public static void main(String ar[])
{
int first,sec,thrd;
first=Integer.parseInt(ar[0]);
sec=Integer.parseInt(ar[1]);
thrd=Integer.parseInt(ar[2]);
System.out.println(” (int first,sec,thrd)first variable is for switch and sec &thrd for calculation”);
switch(first)
{
case 1:
System.out.println(“addition=”+(sec+thrd));
break;
case 2:
System.out.println(“subtraction=”+(sec-thrd));
break;
case 3:
System.out.println(“multiplication=”+(sec*thrd));
break;
case 4:
System.out.println(“divison=”+(sec/thrd));
break;
case 5:
System.out.println(“mod=”+(sec%thrd));
break;
default:
System.out.println(“pls enter numeric value”);
}}}
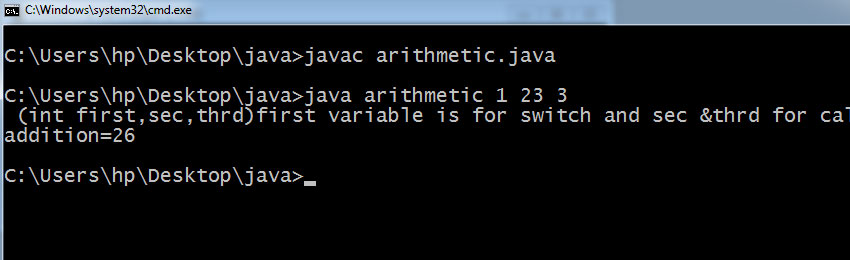
Example 3. Java Program for Addition, subtraction, multiplying and dividing two numbers using a switch(without break)
class arithmetic1
{
public static void main(String ar[])
{
int first,sec,thrd;
first=Integer.parseInt(ar[0]);
sec=Integer.parseInt(ar[1]);
thrd=Integer.parseInt(ar[2]);
System.out.println(” (int first,sec,thrd)first variable is for switch and sec &thrd for calculation”);
switch(first)
{
case 1:
System.out.println(“addition=”+(sec+thrd));
case 2:
System.out.println(“subtraction=”+(sec-thrd));
case 3:
System.out.println(“multiplication=”+(sec*thrd));
case 4:
System.out.println(“divison=”+(sec/thrd));
case 5:
System.out.println(“mod=”+(sec%thrd));
break;
default:
System.out.println(“pls enter numeric value”);
}
}
}
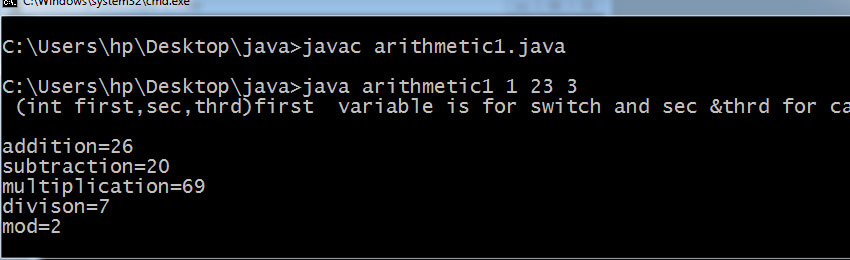