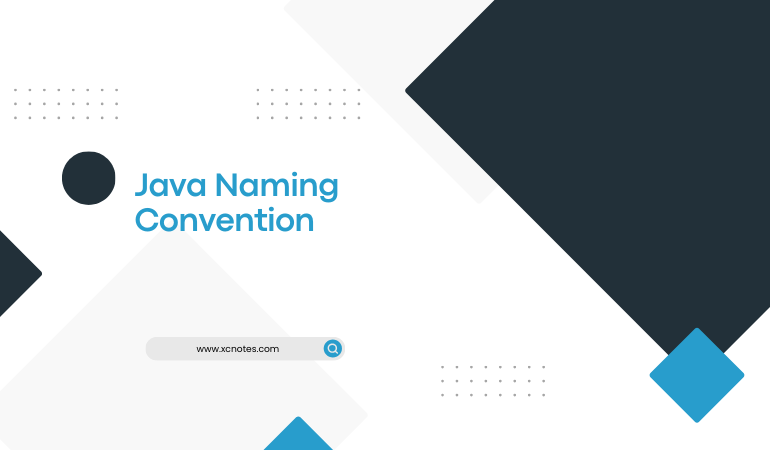
Java Naming Conventions
class JavaNamingConventions
{
static final double PI_VALUE = 3.14;
static int circleRadius = 10;
public static void circleArea()
{
System.out.println(“Circle Area: ” + (PI_VALUE * circleRadius * circleRadius));
}
public static void main(String args[])
{
// method circleArea() is of the static type so we do not need to create an object
circleArea();
}
}
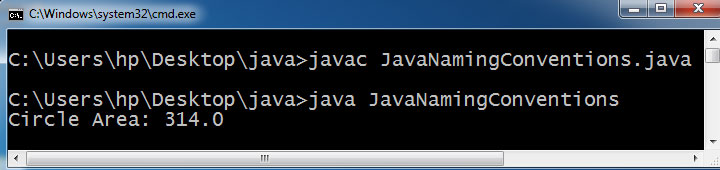
static:
If a variable or method is static in nature then we can access it directly without creating an object.
In the above java program, we used variables, methods, constants, classes, etc. If you notice then the class name is written with the first letter as the capital of each word. Similarly, different naming conventions are followed for constants, variables, and methods.
Using naming conventions makes the program easier to read and understand for you and the other programmer as well.
Before we start with java naming conventions you must know about the camel case as we would be using this term while discussing naming conventions.
CamelCase: CamelCase is a naming convention in which a name is formed of multiple words that are joined together as a single word with the first letter of each of the multiple words as a Capital letter. CamelCase may start with a capital or especially in programming languages a lowercase letter.
For example, Love java programming can be written as LoveJavaProgramming or loveJavaProgramming
Java Naming Conventions For Classes
Classes always start with a capital letter(like Circle) and are CamelCase.
- class Circle
- class VehicleSpecification
- class JavaNamingConventions
Java Naming Conventions For Variables And Methods
Variables and Methods always start with a lowercase letter(like double area) and are camelCase too.
- double area = 10.5;
- int circleRadius = 10;
- circleRadius( );
- rectanglePerimeter( );
Java Naming Conventions For Constants
final instance variables can never change and therefore are constant. Constants are always in capital letters, with any breaks between words represented by an underscore.
- final String TOPIC = “Java Naming Conventions for constant,class,methods and interface “;
- final double PI_VALUE = 3.14;
- final String MY_ENGLISH_TEACHER_NAME = ” Anything which you would like “;
Java Naming Conventions For Packages
Package names are written in all lowercase so as to avoid conflict with the name of classes and interfaces.
- package number;
- package number.integer;
Here integer is the package that is contained in the number package.
Java Naming Conventions For Interfaces
The interface name is capitalized as the class name.
- interface Sorting
- interface RectangleProperties
- interface SortingAndSearching