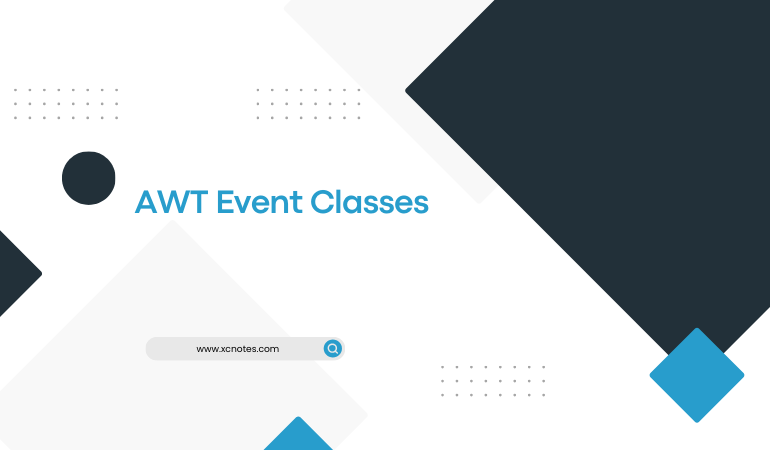
AWT Event Classes
The Event classes represent the events that are generated when an end user interacts with the AWT components. the EventObject class is the superclass for handling all events. the class exists in the package, java. util. The AWTEvent class is the subclass of the EventObject class and all AWT events originate from the AWTEvent class. This class is present in java.awt package.java.awt.event package defines the AWT events derived from the AWTEvent class. The event classes contain costant integer values, which provide information about the events and the state of the components generating events.
Types of Event Classes:
Following are Event Classes used in Java.
- ActionEvent Class:
ActionEvent Class generated Action Event when an end-user clicks a button or a menu option.
- AdjustmentEvent Class:
AdjustmentEvent Class generated Adjustment Event when an end user moves the scrollbar.
3. ComponentEvent Class:
ComponentEvent Class generated Component event when an AWT component is hidden, shown, moved, or resized.
4. FocusEvent Class:
FocusEvent Class generated Focus event when an AWT component gains or loses input focus.
5. ItemEvent Class:
ItemEvent Class generated Item Event when a checkbox, radio button, list item, or choice menu option is selected.
6. KeyEvent Class:
KeyEvent Class generated Key Event when a provides input through the keyboard.
7.MouseEvent Class:
MouseEvent Class class generated Mouse Event when a works with the mouse.
8. MouseWheelEvent Class:
MouseWheelEvent class generated MouseWheelEvent when an end user rotates the mouse wheel.MouseWheelEvent is the subclass of the MouseEvent class.
9.PaintEvent Class:
PaintEvent Class generates Paint Event and paints Event ensuring that the paint() and update() method calls are serialized.
10. TextEvent Class:
TextEvent Class generated text Event when characters are entered in text fields and text area boxes.
11.WindowEvent Class:
WindowEvent Class generated Window Event when an end user activates, deactivates, opens closes, minimizes, or restores a window.
Action Listener is used to the button, the button click event is an example of the Action Listener Interface.ItemListener is used when a CheckBox, RadioButton, ListItem, or Choice menu option is selected. Focus Listener is used when an AWT Component gains or loses input focus.
Event Classes in Java with Example
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class User extends JApplet implements FocusListener,ItemListener,ActionListener
{
//Declaration for data entry controls
JPanel p1;
JLabel l1,l3,l4;
JTextField tf1;
JRadioButton male;
JRadioButton female;
JComboBox c1;
JButton b1;
String subjectarr[]={“Software”,”Movies”,”Literature”,”Society and People”};
String name, sex, subject;
public void init()
{
p1=new JPanel();
getContentPane().add(p1);
l1=new JLabel(“Enter ur Name:”);
l3=new JLabel(“Select sex”);
l4=new JLabel(” ur Interest:”);
tf1=new JTextField(10);
male=new JRadioButton(“Male”);
female=new JRadioButton(“Female”);
c1=new JComboBox(subjectarr);
b1=new JButton(“Submit”);
p1.add(l1);
p1.add(tf1);
p1.add(l3);
p1.add(male);
p1.add(female);
p1.add(l4);
p1.add(c1);
p1.add(b1);
male.addItemListener(this);
female.addItemListener(this);
tf1.addFocusListener(this); //focus listener works on textfield
c1.addActionListener(this);
b1.addActionListener(this);
}
public void itemStateChanged(ItemEvent eev) //for radio button
{
Object obj=eev.getSource(); //in choice we can select only
if(obj==male)
sex=”Male”;
if(obj==female)
sex=”Female”;
if(obj==c1){
subject=String.valueOf(c1.getSelectedItem());
getAppletContext().showStatus(subject);
}
}
public void focusLost(FocusEvent e)
{
Object temp=e.getSource();
if(temp==tf1){
String strName=tf1.getText();
if(strName.length()!=0)
name=strName;
else
getAppletContext().showStatus(“Name is not fill”);
}
}
public void focusGained(FocusEvent e)
{
//Will not be handled
}
public void actionPerformed(ActionEvent e) //action performed on button
{
Object obj=e.getSource();
if(obj==b1)
{
subject=String.valueOf(c1.getSelectedItem());
String recordd=name+” : “+sex+” : “+subject;
getAppletContext().showStatus(recordd); //name, gender,subject show on status of Applet
}
}
}
/*<applet code=”User.class” height=500 width=500></applet>*/
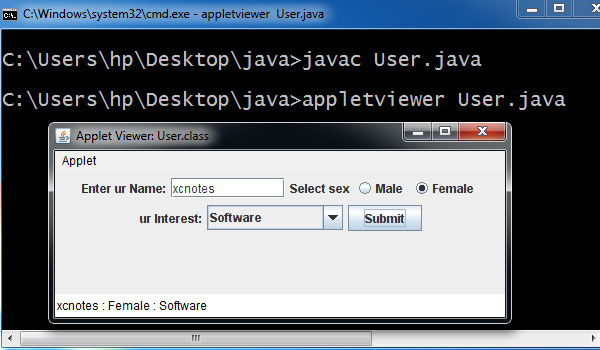
Explanation…
In the above example, we use listeners Action is used for the button(“SUBMIT”), when we click on this button the all the details like the name you enter in the text field, select sex(male, female), and your subject in Choice Box )will show in the status bar of the applet window.