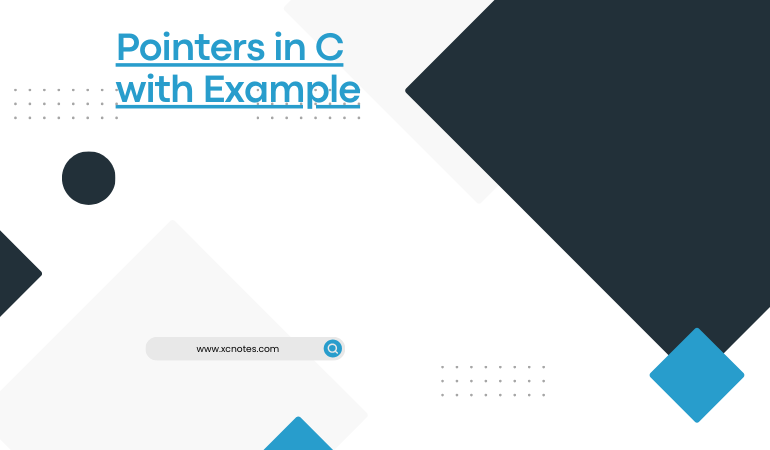
Pointers in C with Example
Introduction of Pointer in C
A pointer is a variable in the C Programming Language that holds the address of another variable of the same data type. For example, an integer variable contains an integer value, however, an integer pointer holds the address of an integer variable. Pointers in C have derived data types. Whenever a variable is declared or initialized in the C programming language, memory is allocated to that variable which stores the value of that variable. We can easily see the address of that variable by using the symbol ‘&’.
Below we have written a program in which we see the address of the variable.
Printing an address of a Variable in C
#include <stdio.h>
int main ( )
{
int a = 12;
printf (“Value of a=%d\n”, a);
printf (“Address of a=%x\n”, &a);
return 0;
}
Output
Value of a =12
Address of a = fff4
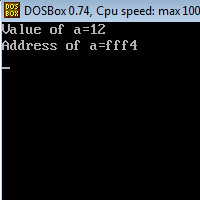
Whenever we declared a variable in the C programming language then memory is allocated to that variable. This memory location has its own address, which we just saw above. Assume that system allocated memory location 5698 for a variable ‘i’.
int i = 12;
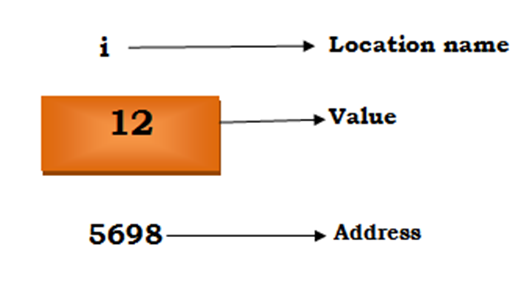
The computer has to assign 5698 memory locations to store the value ’12’. 5698 is not only a number, because some other time the computer may choose a different location for storing the value ’12’. The variable which holds the address is known as the pointer variable.
A pointer variable holds the address of another variable of the same data type and the value of the pointer variable gets stored in the memory location.
Declaration and Initialization of Pointers in C
Declaration of pointer variables
Syntax: datatype *pointer_name;
For example
int *w;
float *x;
char *y;
In int *w, w is an integer data type pointer variable. Similarly, the statement float *x and char *y. Due to the declaration, the compiler allocates memory to the pointer variables w, x, and y. Because no value is assigned to these locations, it contains a garbage value and therefore they point to unknown locations as shown:
int *z;
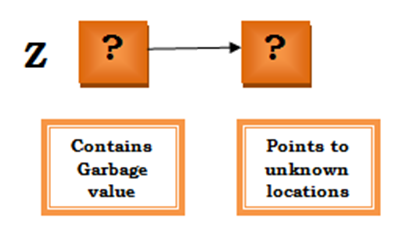
Initialization of pointer variables
When the address of a variable is assigned to a pointer variable then this process is known as initialization. All uninitialized pointers contain some garbage values that will be interpreted as memory addresses. These memory addresses aren’t valid or point to some wrong values and the compiler is unfit to detect or descry these errors(bugs), the program with an uninitialized pointer will give wrong results. So, it’s important to initialize variables in the program very carefully(precisely).
int q; // Variable declaration
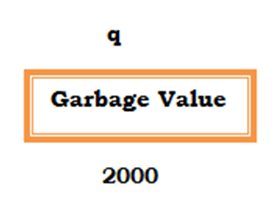
Memory address 2000 is allocated to variable q which consist of a garbage value.
int *p; // Pointer variable declaration
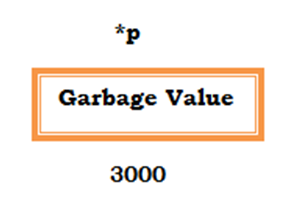
p = &q; // Initialization of pointer variable
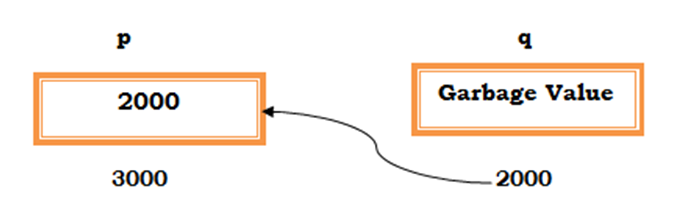
After initialization, pointer variable p holds the address of the variable q which is 2000.
We can also combine the write this type of declaration and initialization
int *p = q;
How to access a variable(int a) through the pointer variable(int *q)?
Once an address of a variable is assigned to a pointer, the question arises that how to access the value of the variable using the pointer? This is done by using a unary operator (*) asterisk. This is also known as the indirection operator or dereferencing operator.
#include <stdio.h>
#include<conio.h>
int main ( )
{
// Declaring variable and pointer variable
int a, *q;
clrscr();
// Initialize variable a
a = 12;
// Initialize pointer variable q
q = &a;
printf (“%d\n”, *q); //This will print the value of a
printf (“%d\n”, *(&a)); // This will print the value of a
printf (“%u\n”, &a); // This will print the address of a
printf (“%u\n”, q); // This will print the address of a
printf (“%u\n”, &q); // This will print the address of q
return 0;
}
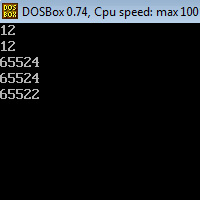
What is a Size of Pointers in C
In the above section, we discussed what is a pointer in C? We will now discuss the size of the pointer and why the size of the pointer changes from one compiler to another compiler. In the manner in which the size of the integer varies from one compiler to another compiler in C programming, the size of the pointer changes from one compiler to another compiler.

The above figure shows that the size of the integer and the size of the pointer varies from compiler to compiler. If the compiler is 16-bit, it occupies 2 bytes, if it’s a 32-bit compiler, it occupies 4 bytes integer and pointer both are same. The reason why the pointer’s size depends on the size of the compiler. 16-bit compiler contains 2^16 memory location.
Total location in the 16-bit compiler would be 2^16 = 65,536.
int a = 134;
The value of the integer 134 will be allocated in memory location between 0 to 65,536.
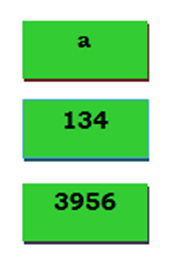
int *ptr;
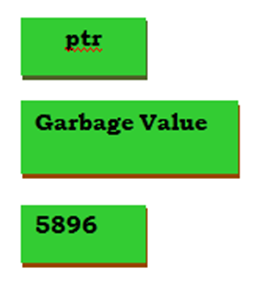
Because the pointer ptr is also the type of integer, the memory location will be allocated between 0 to 65,536.
ptr=&a;
Now, the address of the integer variable ‘a’ was sent to pointer ‘ptr’.
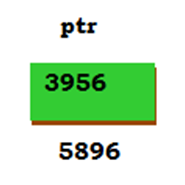
Similarly, the whole process is for 32-bit and 64-bit compiler.
C program to calculate the size of pointers.
#include <stdio.h>
#include <conio.h>
int main ( )
{
int a = 32;
char ch = ‘d’;
int *b;
char *cp;
b = &a;
cp = &ch;
printf (“size of pointer b is %d and cp is %d\n”, sizeof(b), sizeof(cp));
return 0;
}
You all might be thinking that its output will be 1 byte for character pointer ‘cp’ and 4 bytes for the integer pointer ‘b’. But this is not true, both the character pointer and the integer pointer will have the size of 4 bytes. Why do so, let’s talk about it.
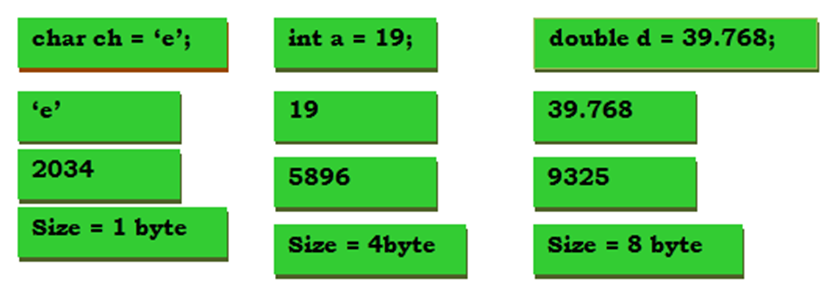
Whenever you declare an ordinary variable, size varies from one data type to another type because the type of data you are storing is different.
But, when we are declaring character pointer, integer pointer and float pointer holding the address of variable ‘ch’, ‘a’ and ‘d’.
char *c = &ch;
i.e. character pointer ‘c’ storing address 2034.
int *b = &a;
and integer pointer ‘b’ storing address 5896.
double *w = &d;
and it holds the address 9325.
Using pointer variables always we are storing the same type of data. All pointer variables hold the address of an ordinary variable, it is not holding the data, so, what it is holding? It is holding the address 2034, 5896, and 9325. Pointer variable always holds the address, so, it always occupies 2 bytes of memory. Pointer size is always fixed because it is holding addresses instead of data.