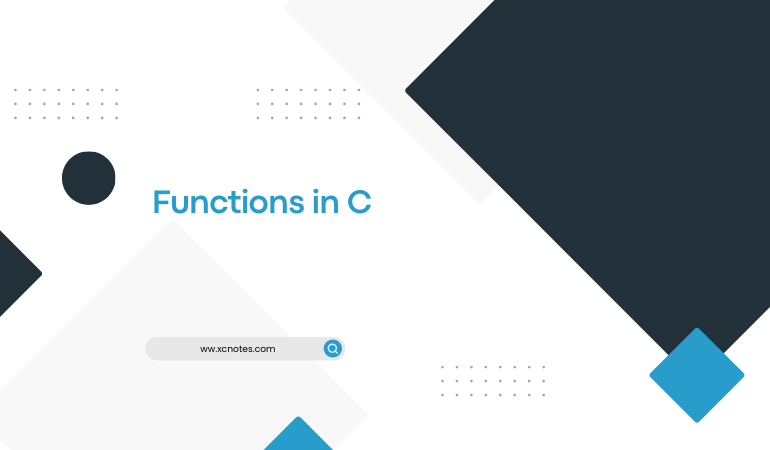
Functions in C
In C programming language, a function is a self-contained block of code that performs a specific task. Functions are used to break down a program into smaller, manageable pieces(chunks), making the code more modular(small parts), reusable(code reusable), and easier to understand and maintain.
C functions have the following components:
Function Declaration: It specifies the function’s name, return type, and parameters (if any). For example:
int add(int a, int b);
.Function Definition: It contains the actual implementation of the function, including the code block enclosed within curly braces {}. For example:
int add(int a, int b) {
int sum = a + b;
return sum;
}
Function Call: It is the point in the program where the function is invoked or called to perform its task. For example:
int result = add(2, 3);
C functions can be classified into several types based on their characteristics:
Standard Library Functions: These are built-in functions provided by the C standard library, such as printf(), scanf(), strlen(), etc. They are readily available and can be used without any additional setup.
User-defined Functions: A user-defined function is a function(method) that is created by the programmer(user) to perform a specific task or set of tasks(single task or group of tasks). It allows you to encapsulate a block of code that can be reused multiple times within a program, promoting modularity and code reusability.
Library Functions: These are functions provided by external libraries or header files that extend the functionality of the C language. Examples include functions from the math library (pow(), sqrt()), string handling library (strcpy(), strcat()), and many others.
Parameterized Functions: These functions take one or more parameters (input values) that are passed to them when they are called. Parameters allow functions to work with different data values each time they are called.
Void Functions: These functions do not return a value. They are used when the task is to be performed without returning any result.
Function with Return Value: These functions return a value as a result of their execution. The return type of such functions can be any valid data type in C.
These are the common types of functions in C. Each type serves a specific purpose and can be used based on the requirements of the program.
Built-In-Functions
C language provides several built-in functions that can be used to perform various operations. Here are some commonly used functions in C:
printf(): Used to print output to the standard output (usually the console).
scanf(): Used to read input from the standard input (usually the console).
strlen(): Calculates the length of a string.
strcpy(): Copies one string to another.
strcat(): Concatenates (joins) two strings.
strcmp(): Compares two strings and returns an integer indicating their relative order.
atoi(): Converts a string to an integer.
srand(): Seeds the random number generator.
rand(): Generates a pseudo-random number.
abs(): Calculates the absolute value of an integer.
pow(): Calculates the power of a number.
sqrt(): Calculates the square root of a number.
ceil(): Rounds a floating-point number up to the nearest integer.
floor(): Rounds a floating-point number(like 2.45) down to the nearest integer.
getchar(): Reads a single character(like ‘r’) from the standard input.
putchar(): Writes a single character(like ‘s’) to the standard output.
toupper(): Converts a character to uppercase.
tolower(): Converts a character to lowercase.
exit(): Terminates the program execution.
These are just a few examples of the built-in functions available in C. C also allows you to define your own functions to perform specific tasks.
Example of Built-in-function in C
#include <stdio.h>
#include <math.h> // Include the math.h header for math functions
#include<conio.h>
void main() {
int num = 16;
// Square root function
double squareRoot = sqrt(num);
int value = -10;
int absoluteValue = abs(value);
int base = 2;
int exponent = 5;
double powerResult = pow(base, exponent);
clrscr();
printf(“Square root of %d = %.2f\n”, num, squareRoot);
// Absolute value function
printf(“Absolute value of %d = %d\n”, value, absoluteValue);
// Power function
printf(“%d raised to the power %d = %.2f\n”, base, exponent, powerResult);
getch();
}
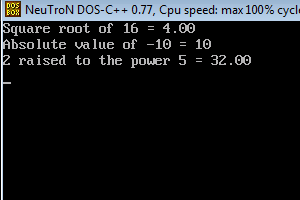
User Defined Functions
In C programming, you can define your own functions to perform specific tasks. Here’s the general structure of a user-defined function:
return_type function_name(parameter_list) {
// Function body or implementation
// Code statements
// Return statement (if applicable)
}
Let’s break down the components of a user-defined function:
return_type: It specifies the data type of the value the function(ex.int fun()) returns. It can be any valid C data type, including void if the function does not return any value.
function_name: It is the name given to the function, which should follow the rules for naming identifiers in C. The function name should be unique within the scope of the program.
parameter_list: It specifies the input parameters (if any) required by the function. Each parameter consists of a data type (like (int a,float b))and a parameter name. Parameters are optional, and you can have multiple parameters separated by commas.
Function body or implementation: It contains the actual code statements that define the behavior and logic of the function. This block of code is enclosed within curly braces {}.
Return statement (if applicable): If the function has a return type other than void, it should include a return statement to specify the value to be returned. The return statement can be placed anywhere within the function body.
Here’s an example of a user-defined function that calculates the sum of two integers:
int sum(int a, int b) {
int result = a + b;
return result;
}
In the above example, sum is the function name, int is the return type indicating that the function returns an integer value, and a and b are the parameters of type int. The function calculates the sum of a and b( int result = a + b;) and returns the result using the return statement.
You can call the user-defined function in your program by using its name and providing the necessary arguments:
int main() {
int x = 42, y =23;
int res = sum(x, y);
printf(“The sum is: %d\n”, res);
return 0;
}
In this example, the sum function is called with arguments x and y, and the result is stored in the variable res. The value of res is then printed using the printf function.
Example of User-define Function in C
#include<stdio.h>
#include<conio.h>
int a,b,c; //variable declaration
//function prototype
void f1();
int f2();
void f3(int);
int f4(int,int);
void main() //main method
{
clrscr();
//function calling
f1();
printf(“\n%d”,f2());
f3(2);
printf(“\n%d”,f4(2,3));
getch();
}
void f1() //without arguments without return
{
a=8;
b=6;
printf(“\nsum=%d”,(a+b));
}
int f2() //without arguments with return
{
return(a-b);
}
void f3(int x) //with arguments without return
{
if(x>0)
printf(“\npositive”);
else
printf(“\nnegative”);
}
int f4(int x,int y) //with arguments with return
{
if(x>y)
return(x);
else
return(y);
}
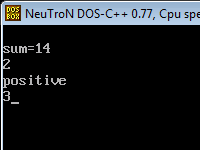