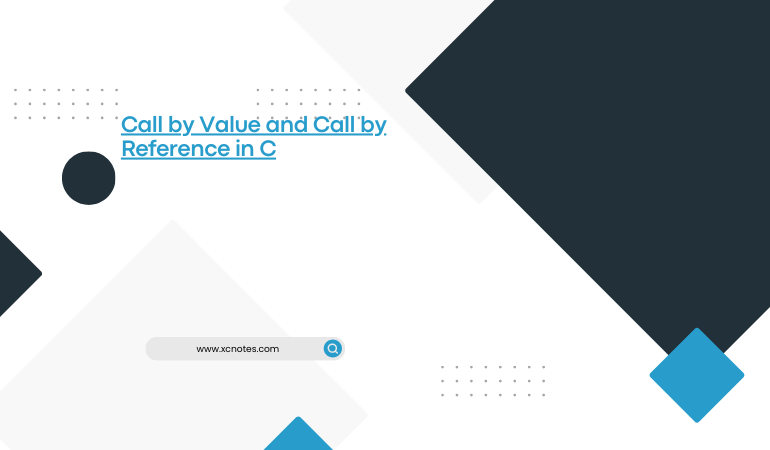
Call by Value and Call by Reference in C
Argument passing (change(a,b), change(x,y))is a technique for transferring data and information between the calling and called function. There are two ways to pass arguments to the called function: a)Call by Value b)Call by reference.
Call by Value: The call by value method is the default technique for passing arguments. In this method only the copies of the caller’s variables are sent to the callee’s variables means a photocopy of actual arguments is passed to called function.
Call by Value in C
#include<stdio.h>
#include<conio.h>
void change(int,int); //prototype
void main()
{
int a=100,b=200;
clrscr();
change(a,b); //function calling
printf(“\na=%d b=%d”,a,b);
getch();
}
void change(int x,int y) //function
{ //function body
int t;
t=x;
x=y;
y=t;
printf(“\nx=%dy=%d”,x,y);
}
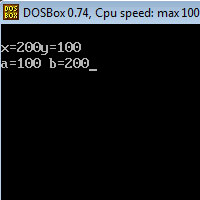
Call by Reference in C
When the arguments listed in a function call are pointers, the memory address of the caller’s variables is transmitted to the function means the address of actual arguments of the calling function is copied into formal arguments(change(int *x, int *y)) of the called function.
#include<stdio.h>
#include<conio.h>
void change(int *,int *); //prototype
void main()
{
int a=100,b=200;
clrscr();
change(&a,&b); //calling function with actual arguments
printf(“\na=%d b=%d”,a,b);
getch();
}
void change(int *x,int *y)
{
int *t;
*t=*x;
*x=*y;
*y=*t;
printf(“\nx=%dy=%d”,*x,*y);
}
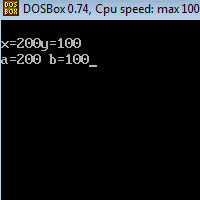
Explanation:
Three things that must be kept in mind while passing arguments are:
1)Number of arguments in the caller and callee function must be the same.
2)Order of the arguments in the caller and callee function should match.
3)The data type of actual and formal arguments should be the same in both the caller and callee function. Any mismatch in the data type will produce unexpected results.
4) & address of operator * value of operator
social media strategy for nonprofits
I would like to thnkx for the efforts you have put in writing this blog. I am hoping the same high-grade blog post from you in the upcoming as well. In fact your creative writing abilities has inspired me to get my own blog now. Really the blogging is spreading its wings quickly. Your write up is a good example of it.