In C++, the “this” pointer is a keyword that represents a pointer to the instance of the class to which the member function belongs. It is implicitly passed as a hidden argument to all member function calls.
Here’s a basic explanation of the “this” pointer:
Types of this pointer
Pointer to the Current Object:
Inside a non-static member function of a class, the “this” pointer points to the object for which the member function is called.
Usage in Member Functions:
When you access a member variable or call a member function inside a class, the compiler uses the “this” pointer implicitly to resolve the object.
class MyClass {
public:
void printAddress() {
// “this”(keyword) is a pointer to the current object
cout << “Address of current object: ” << this << endl;
}
};
Necessary in Ambiguous Situations:
It becomes especially useful when there is a need to distinguish between member variables and local variables with the same name.
class MyClass {
private:
int x;
public:
void setX(int x) {
// Use “this” to refer to the member variable
this->x = x;
}
};
Implicit Usage:
In most cases, you don’t need to explicitly use the “this” pointer, as it’s automatically provided by the compiler.
MyClass obj;
obj.printAddress(); // “this” is automatically passed by the compiler
Not Available in Static Member Functions:
Since static member functions don’t operate on a specific instance of the class, they don’t have a “this” pointer.
class MyClass {
public:
static void staticFunction() {
// No “this” pointer in static functions
}
};
Understanding and using the “this” pointer is fundamental when working with C++ classes, especially in situations where the distinction between member variables and local variables is necessary.
C++ THIS KEYWORD EXAMPLE
#include<iostream.h>
#include<conio.h>
class rectangle
{
private:
int l,b;
public:
void set_data(int i,int j)
{
cout<<“address~generated call set-data”<<this<<“\n”;
this->l=i;
this->b=j;
}
void area()
{
cout<<“the address generated call the area”<<this<<“\n”;
cout<<this->l*this->b; //multiply l*b
}};
void main()
{
rectangle r1;
clrscr();
r1.set_data(5,6);
r1.area();
getch();
}
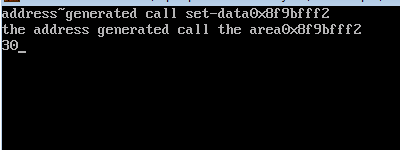