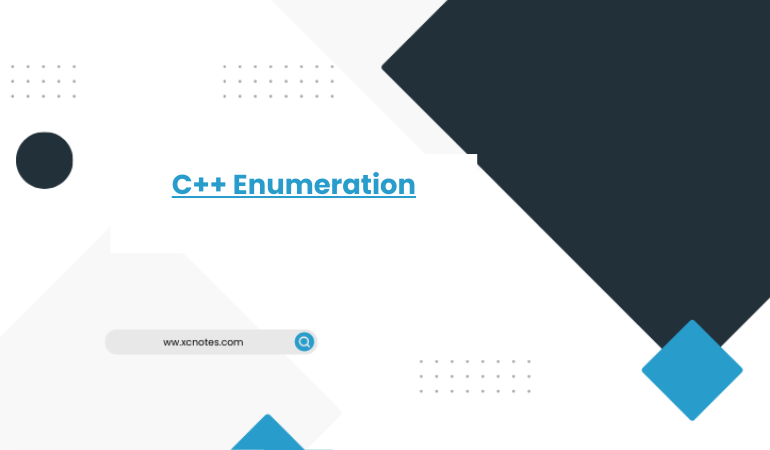
C++ Enumeration
In C++, enumeration (enum) is a user-defined data type that consists of named integral constants. Enums are useful for creating more readable and self-explanatory code by assigning names to numeric values.
Here’s a basic example of how to use enums in C++:
#include <iostream.h>
// Define an enumeration named Color
enum Color {
RED, // 0
GREEN, // 1
BLUE // 2
};
int main() {
// Declare a variable of type Color
Color myColor = RED;
// Use the variable
if (myColor == RED) {
cout << “The color is red.” << endl;
} else if (myColor == GREEN) {
cout << “The color is green.” << endl;
} else if (myColor == BLUE) {
cout << “The color is blue.” << endl;
}
return 0;
}
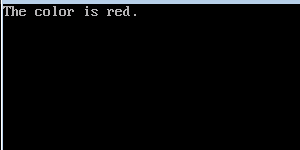
In this example, the Color enum has three named constants: RED, GREEN, and BLUE. By default, these constants are assigned integral values starting from 0. You can explicitly assign values to enum constants if needed.
Enums are often used to improve code readability and maintainability by giving meaningful names to numeric values. They are commonly used in switch statements, function parameters, and other situations where a set of related constant values is required.
C++ Enumeration example
#include <iostream.h>
// Define an enumeration named Day
enum Day {
SUNDAY, // 0
MONDAY, // 1
TUESDAY, // 2
WEDNESDAY, // 3
THURSDAY, // 4
FRIDAY, // 5
SATURDAY // 6
};
// Function that takes a Day enum as a parameter
void printDay(Day day) {
switch (day) {
case SUNDAY:
cout << “Sunday” << endl;
break;
case MONDAY:
cout << “Monday” << endl;
break;
// … (cases for other days)
default:
cout << “Invalid day” << endl;
break;
}
}
int main() {
// Use the function with an enum parameter
printDay(WEDNESDAY);
return 0;
}
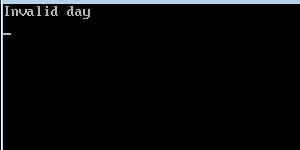
In this example, the Day enum is used to represent the days of the week, and the printDay function demonstrates how to use enums in switch statements.
Run
Alt+f9
Ctr+f9
Alt+f5