Write a program in Java to Swap two digits using a third variable.
import java.util.Scanner;
class Swapping
{
public static void main(String args[])
{
int first_no, sec_no, third_no;
System.out.println(“Enter first_no and sec_no”);
Scanner in = new Scanner(System.in);
first_no = in.nextInt();
sec_no = in.nextInt();
System.out.println(“Before Swapping\nfirst_no = “+first_no+”\nsec_no = “+sec_no);
third_no = first_no;
first_no = sec_no;
sec_no = third_no;
System.out.println(“After Swapping\nfirst_no = “+first_no+”\nsec_no = “+sec_no);
}
}
The above program shows sec_no how to swap two digits using a third variable. In this program, we have three variables first_no,sec_no, and third_no. We accept values of first_no and sec_no from the user. We use the third_no variable as a third variable which is used to store the first_no variable value. Then swapping is sec_no as shown in the above program.
Output:
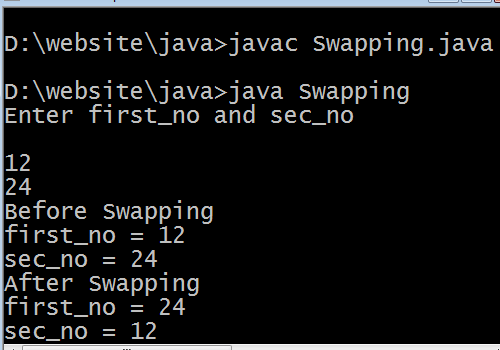
Write a program in Java to Swap two digits Without using a third variable.
import java.util.Scanner;
class Swapping1
{
public static void main(String args[])
{
int first_no, sec_no;
System.out.println(“Enter first_no and sec_no”);
Scanner in = new Scanner(System.in);
first_no = in.nextInt();
sec_no = in.nextInt();
System.out.println(“Before Swapping\nfirst_no = “+first_no+”\nsec_no = “+sec_no);
first_no=first_no+sec_no;
sec_no=first_no-sec_no;
first_no=first_no-sec_no;
System.out.println(“After Swapping\nfirst_no = “+first_no+”\nsec_no = “+sec_no);
}
}
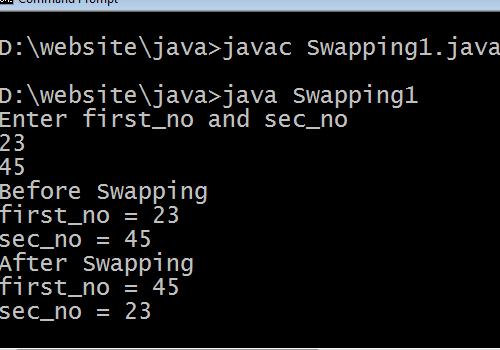
The above program shows sec_no how to swap two digits without using a third variable. In this program, we have two variables first_no and sec_no. we accept values of first_no and sec_no from the user.