This Java program source code converts temperature from Fahrenheit to Celsius scale.
import java.util.*;
class FahrenheitCelsius
{
public static void main(String[] args)
{
float temp;
Scanner inn = new Scanner(System.in);
System.out.print(“Enter temperature in Fahrenheit=”);
temp= inn.nextInt();
temp = ((temp – 32)*5)/9;
System.out.println(“Temperature in Celsius = ” + temp);
}
}
In the above program, you enter a temperature in Celsius and it will show its equivalent in Fahrenheit temperature. temp variable is used for temperature. We use Scanner classes. This is a straightforward program but it’s useful to demonstrate input with Scanner Class and output with System.out.println.
The output of the above program is:
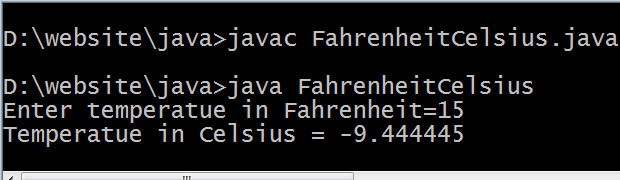
For Celsius to Fahrenheit conversion, we use the formula
T = 9*T/5 + 32
where T is the temperature on the Celsius scale.