Java program to calculate rectangle area using Scanner classes:
import java.util.Scanner;
public class Rarea
{
public static void main(String[] args)
{
Scanner inn = new Scanner(System.in);
double height, width, area;
System.out.println(“Area of Rectangle”);
System.out.print(“Please enter the height: “);
height = inn.nextDouble();
System.out.print(“Please enter the width: “);
width = inn.nextDouble();
area = height * width;
System.out.println(“Area: ” + area);
}
}
In above program, the user enters the width and height of the rectangle. The rectangle area can be found by multiplying the width by the height.
The formula for the area is:
Area = width * height
the output of the above program is:

Area:600.0 result is shown in floating points because in the program we declare the variable width, height, and area as double. we use double data types. for example, if we have a double value then
Java program to calculate rectangle area without using Scanner classes:
class Rarea1 {
public static void main (String[] args)
{
double length = 22.3;
double width = 24.6;
double area = length*width;
System.out.println(“Area of Rectangle is:”+area);
}
}
the output of the above program is:
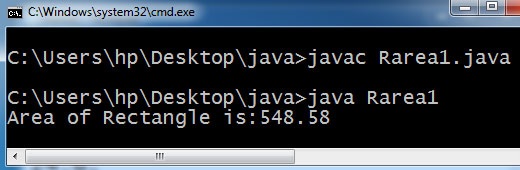