An Armstrong number is a number(narcissistic number) equal to the sum of its own digits raised to the power of the number of digits. Here’s an example of how you can check if a number is an Armstrong number in Java:
import java.util.Scanner;
public class ArmstrongNumber {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter a number: “);
int number = scanner.nextInt();
if (isArmstrong(number)) {
System.out.println(number + ” is an Armstrong number.”);
} else {
System.out.println(number + ” is not an Armstrong number.”);
}
}
public static boolean isArmstrong(int number) {
int originalNumber = number;
int numDigits = String.valueOf(number).length();
int sum = 0;
while (number != 0) {
int digit = number % 10;
sum += Math.pow(digit, numDigits);
number /= 10;
}
return sum == originalNumber;
}
}
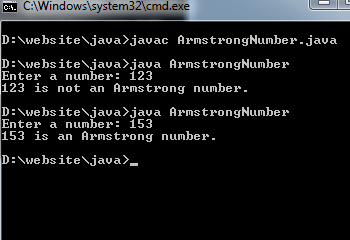
In this code, the first user enters a number. Then, we call the isArmstrong() method to check if the entered number is an Armstrong number. The isArmstrong() method calculates the sum of each digit raised to the power of the number of digits and checks if it equals the original number.
You can run this code, enter a number, and it will tell you whether the number is an Armstrong number or not.
Armstrong number is that number which of three integer digits, which sum of the cube
of its individual digits(like 13+53+33) is equal (=)to that number which we enter like 153, the cube of 1 and the cube of 5, and the cube of 3 and the sum of both (1*1*1)+(5*5*5)+(3*3*3)=153 means its Armstrong number.
others are…..370,371,407 etc
Armstrong Number Example in Java
class arm
{
public static void main(String aa[])
{
int number,sum=0,temp,remainder;
number=Integer.parseInt(aa[0]); //number enter by user(run time0
temp=number; //value assign to variable temp
while(temp!=0) //condition check if temp is not equal 0 then
{
remainder=temp%10; //show remainder
sum=sum+remainder*remainder*remainder; //calculation
temp=temp/10;
}
if(number==sum) //if the value of the number and the sum are equal then Armstrong
System.out.print(“armstrong”);
else
System.out.print(“not”);
}}
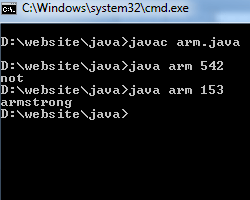
Explanation…
In the above example, we enter the value(integer type). If the value is not equal to zero then the
calculation will start. After analysis it checks the output if the number and the sum are equal then
the result will be Armstrong otherwise not.