A circular linked list is a type of linked list where the last node of the list points back to the first node instead of having a null pointer. In other words, the next pointer of the last node in the list points to the head of the list, creating a circular structure.
Circularly linked lists have a variety of applications. For example, they can be used to implement circular buffers, where data can be continuously read and written in a loop. They are also useful in solving problems that involve circular movement, such as iterating over a set of elements repeatedly.
To create a circular linked list, you need to ensure that the last node’s next pointer is set to the first node instead of null. When traversing or performing operations on a circular linked list, you should take into account the circular nature to avoid infinite loops.
A circular linked list is a variation of a linked list in which the last node points back to the first node instead of pointing to null. This creates a circular structure where the elements are connected in a circular fashion.
In a traditional linked list, the last node’s next pointer typically points to null to indicate the end of the list. However, in a circular linked list, the last node’s next pointer points back to the first node, completing the circle.
Node A -> Node B -> Node C -> Node D
^ |
| |
——————————————–
his example, Node D points back to Node A, forming a circular structure.
To implement a circular linked list, you can use a regular linked list structure with a modification to ensure the last node points to the head.
A linear linked list in which the last element points to the first element(The Link field of the last node always points to the starting node of the list), thus, forming a circle.
/* Circular linked list Example*/
# include <stdio.h>
# include <malloc.h>
struct nodee
{
int infoo;
struct nodee *linkk;
}*lastt;
main()
{
int choicee,no,mm,poo,ii;
lastt=NULL;
while(1)
{
printf(“Press 1…Create List\n”);
printf(“Press 2…Add at begining\n”);
printf(“Press 3…Add after \n”);
printf(“Press 4…Delete\n”);
printf(“Press 5…Display\n”);
printf(“Press 6…Quit\n”);
printf(“Enter your choicee : “);
scanf(“%d”,&choicee);
switch(choicee)
{
case 1:
printf(“Enter the node……> : “); /* to be created*/
scanf(“%d”,&no);
for(ii=0; ii < no;ii++)
{
printf(“Enter the data(number) value…….> : \n”);
scanf(“%d”,&mm);
create_list(mm);
}
break;
case 2:
printf(“Enter the number(for beginning) …….> : “);
scanf(“%d”,&mm);
addatbeg(mm);
break;
case 3:
printf(“Enter the number(for any position)……..> : “);/*(any position)*/
scanf(“%d”,&mm);
printf(“Enter the poosition……>\n “);/*(after which this data value is inserted)*/
scanf(“%d”,&poo);
addafter(mm,poo);
break;
case 4:
if(lastt == NULL)
{
printf(“Oops! List underflow\n”);
continue;
}
printf(“Enter the any(for deletion) one number……>:\n “);/*to be deletion*/
scanf(“%d”,&mm);
del(mm);
break;
case 5:
display();
break;
case 6:
exit();
default:
printf(“Wrong choicee\n”);
}/* switch End*/
}/* while End*/
}/* main() End*/
create_list(int numm)
{
struct nodee *qq,*tmpp;
tmpp= malloc(sizeof(struct nodee));
tmpp->infoo = numm;
if(lastt == NULL)
{
lastt = tmpp;
tmpp->linkk = lastt;
}
else
{
tmpp->linkk = lastt->linkk; /*added at the end of list*/
lastt->linkk = tmpp;
lastt = tmpp;
}
}/* create_list() End*/
addatbeg(int numm)
{
struct nodee *tmpp;
tmpp = malloc(sizeof(struct nodee));
tmpp->infoo = numm;
tmpp->linkk = lastt->linkk;
lastt->linkk = tmpp;
}/* addatbeg() End*/
addafter(int numm,int poos)
{
struct nodee *tmpp,*qq;
int ii;
qq = lastt->linkk;
for(ii=0; ii < poos-1; ii++)
{
qq = qq->linkk;
if(qq == lastt->linkk)
{
printf(“There are less than %d elements\n”,poos);
return;
}
}/* for End*/
tmpp = malloc(sizeof(struct nodee) );
tmpp->linkk = qq->linkk;
tmpp->infoo = numm;
qq->linkk = tmpp;
if(qq==lastt) /*Element inserted at the end*/
lastt=tmpp;
}/* addafter() End*/
del(int numm)
{
struct nodee *tmpp,*qq;
if( lastt->linkk == lastt && lastt->infoo == numm) /*Only one element*/
{
tmpp = lastt;
lastt = NULL;
free(tmpp);
return;
}
qq = lastt->linkk;
if(qq->infoo == numm)
{
tmpp = qq;
lastt->linkk = qq->linkk;
free(tmpp);
return;
}
while(qq->linkk != lastt)
{
if(qq->linkk->infoo == numm) /*Element deleted in between*/
{
tmpp = qq->linkk;
qq->linkk = tmpp->linkk;
free(tmpp);
printf(“%d deleted\n”,numm);
return;
}
qq = qq->linkk;
}/* while End*/
if(qq->linkk->infoo == numm) /*lastt element deleted qq->linkk=lastt*/
{
tmpp = qq->linkk;
qq->linkk = lastt->linkk;
free(tmpp);
lastt = qq;
return;
}
printf(“Element %d not found\n”,numm);
}/* del() End*/
display()
{
struct nodee *qq;
if(lastt == NULL)
{
printf(“List is empty\n”);
return;
}
qq = lastt->linkk;
printf(“List is :\n”);
while(qq != lastt)
{
printf(“%d “, qq->infoo);
qq = qq->linkk;
}
printf(“%d\n”,lastt->infoo);
}/* display() End*/
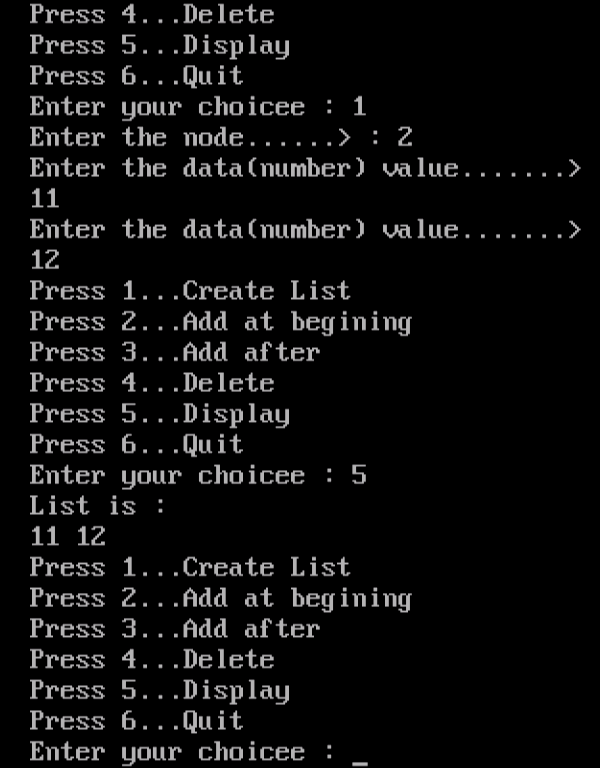