Strings in C are the collection of characters. Strings are also known as an “Array of Characters”.
Strings in C is a one-dimensional array.
Strings in C are ended by the “NULL” (‘\0’) character.
X | C | N | O | T | E | S | \0 |
Strings are written in double quotes (“Xcnotes”) while the character is written inside a single quote (‘X’).
In C programming, strings are not used as a data types (like char(for character), float(for decimal value like 6.78) , double(for decimal like 6.789), int(for integer value like 5,7) etc.).
Declaration of Strings in C
Strings are declared like an array(char your_name[20]) if you do not know what the arrays are? Then, first, you should read the arrays(User define, Non-primitive data type).
What is Array in C?
1. char str[5];
If we write char as “str[5]” then 5 bytes of memory are reserved for ‘str’.
2. char str[ ];
If we write char as “str[ ]” then the memory is allocated at the time of the program’s execution.
Initialization of Strings in C
Strings in C can be initialized in many ways,
Way 1
char str[8] = “Xcnotes”;
or
char str[ ] = “Xcnotes”;
In the above initialization, NULL (‘\0’) character is automatically inserted at the end of the string.
Way 2
char str[8] = {‘X’, ‘C’, ‘N’, ‘O’, ‘T’, ‘E’, ‘S’, ‘\0’};
or
char str[ ] = {‘X’, ‘C’, ‘N’, ‘O’, ‘T’, ‘E’, ‘S’, ‘\0’};
str[0] | str[1] | str[2] | str[3] | str[4] | str[5] | str[6] | str[7] |
X | C | N | O | T | E | S | \0 |
Representation of string in a one-dimensional form
str[0] | str[1] | str[2] | str[3] | str[4] | str[5] | str[6] | str[7] |
X | C | N | O | T | E | S | \0 |
2000 | 2001 | 2002 | 2003 | 2004 | 2005 | 2006 | 2007 |
2000 |
A string variable ‘str’ always holds the base address of a character array. It is also known as internal pointers.
C program in which a string is declared and printed.(String in C Example)
#include <stdio.h>
int main()
{
char str[8]=”Program”;
char str1[8] = {‘P’,’r’,’o’,’g’,’r’,’a’,’m’,’\0′};
printf (“String Literal is : %s\n”, str);
printf (“Character array is :%s\n”, str1);
return 0;
}
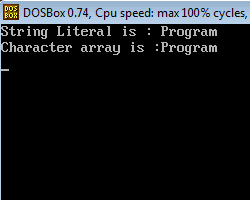
Read String from the user in C
‘Scanf’ function is used to read strings in C from the user.
‘Scanf’ function reads strings continuously until whitespace occurs.
Example
#include <stdio.h>
int main()
{
char str[10];
printf (“Enter String”);
scanf (“%s”, str);
printf (“String is %s\n”, str);
return 0;
}
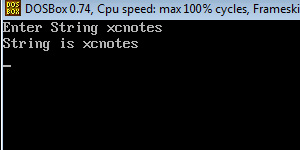
If whitespace is given in the input then scanf will read the string coming from the first whitespace. You can read this in the given program below,
#include <stdio.h>
int main()
{
char str[30];
printf (“Enter String”);
scanf (“%s”, str);
printf (“String is %s\n”, str);
return 0;
}
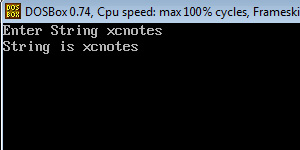
If you want to read strings given with whitespace, then use the gets ( ) function and this function is available in ‘stdio.h’ header file.
C program gets() and puts() function
#include <stdio.h>
int main()
{
char str[30];
printf (“Enter String\n”);
gets (str);
printf (“string is %s \n”, str);
return 0;
}
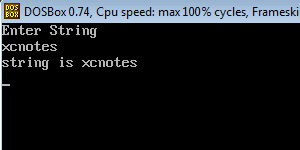
puts( ) function is used to print the output on the screen.
#include <stdio.h>
#include <conio.h>
int main()
{
char str[10];
printf (“Enter string\n\t”);
gets(str);
puts(str);
return 0;
}
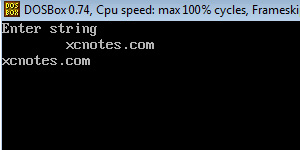
Library String Functions in C with Example
‘String.h’ header file consists of lots of library function like, strlen( ), strcpy( ), strcmp( ), strcat(), strrev( ) etc.
strlen | Use for Find the length of the String(xcnotes (6 lengths) |
strcat | Appends 2 strings(Concat 2 strings) |
strcpy | Copy the string |
strcmp | Compare 2 strings |
strrev | Reverse the string(SETONCX) |
strupr | Convert string into Upper case(XCNOTES) |
strlwr | Convert the string into Lower case(xcnotes) |
Strlen() function in C
Strlen ( ): This function calculates the length of string excluding null (‘\0’) character.
Syntax:
Strlen (Name_of_string);
Program to calculate the length of the Strings in C
#include <stdio.h>
#include <string.h>
int main()
{
int length;
char str[]=”XCNOTES”;
length = strlen(str);
printf (“Length of string is = %d\n”,length);
return 0;
}
Output
The length of the string is 7
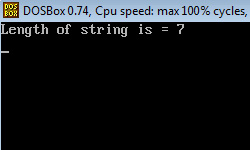
Strcat() Function in C
Strcat( ): This function connects two strings together.
Syntax
strcat (string1, string2);
Program to concatenates two strings in C.
#include <stdio.h>
#include <string.h>
int main()
{
char str1[]=”Xcnotes”;
char str2[]=”.co”;
strcat(str1,str2);
printf(“Concatenate string is %s”, str1);
return 0;
}
Output
Concatenate String is Xcnotes.co
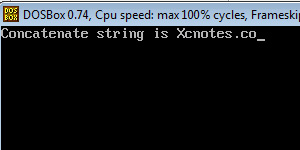
Strcmp() function in C
strcmp ( ): This function compares two strings. If the two strings are equal then it returns 0 otherwise it returns 1.
Syntax
strcmp (string1, string2);
When string1 is greater than string2, then it returns 1.
When string1 is less than string2, then it returns -1.
If string1 and string2 both are equal then strcmp ( ) function returns zero.
Program to compare two strings in C.
#include <stdio.h>
#include <string.h>
int main()
{
char str1[]=”come”;
char str2[]=”Welcome”;
int w,x,y,z;
w = strcmp(str1, “come”);
x = strcmp(str1, “Welcome”);
y = strcmp(str1,”f”);
z = strcmp(str1,”feel”);
printf (“w=%d\n x=%d\n y=%d\n z=%d\n”, w,x,y,z);
return 0;}
Output:
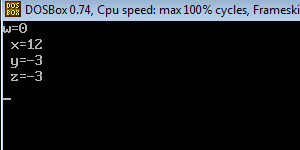
strcpy() function in C
Strcpy( ): This function moves or copies the content of one string into another string.
Syntax
strcpy (string1, string2);
Program to copy the content of one string to another string in C.
#include <stdio.h>
#include <string.h>
int main()
{
char str1[] = “DIGITAL”;
char str2[] = “INDIA”;
strcpy(str1,str2);
printf(“Final String = %s\n”, str1);
return 0;
}
Output
Final string = INDIA
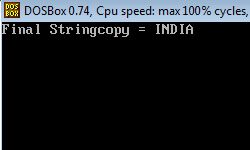
Note: If the length of the destination string is less than the source string then the source string is not copied into the destination string.
Strchr() Function in C
strchr ( ): This function helps you to find the first occurrence of the desired character in a string.
Syntax
strchr ( string, i);
Program to find the desired character in a string.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”The C programming languages”;
char *ptr;
ptr = strchr (str,’m’);
printf (“String in which first character is occur = %s\n”, ptr);
return 0;
}
Output
String in which the first character is occurring =mming languages
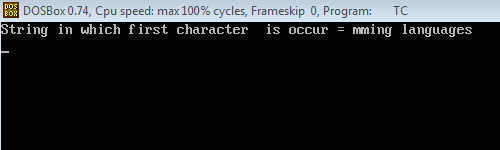
Strrchr()function in C
Strrchr ( ): This function tells about the last occurrence of the desired character in a string.
Syntax
strrchr(Name_of_string, ‘Desired Character);
Program to find out the last occurrence of the desired character in a string.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”The C programming language”;
char *ptr;
ptr = strrchr(str,’i’);
printf (“The Last occurence of character in a string = %s\n”, ptr);
return 0;
}
Output
The last occurrence of the character in a string = ing language
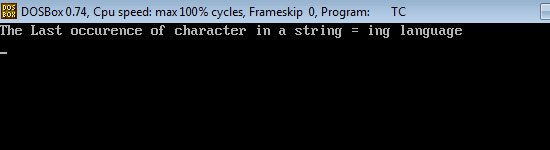
Strstr() function in C
Strstr ( ): With the help of this function we can find the desired string in the given string.
Syntax
strstr(Name_of_string, “desired string”);
C Program to find the desired string in given string.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”the c programming language”;
char *ptr;
ptr = strstr (str, “programming”);
printf (“First occurrence of string is =%s\n”, ptr);
return 0;
}
Output
The first occurrence of the string is = programming language.
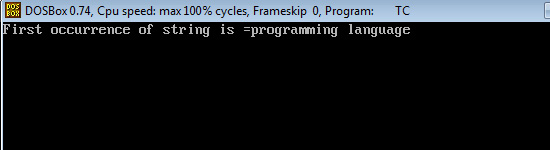
Strlwr() function in C
Strlwr ( ): This function converts a given string into lower case.
Syntax
strlwr (string);
C program to convert the string in to lower case.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”MANGO SEASON IS BACK”;
printf(“%s\n”,strlwr(str));
return 0;
}
Output
mango season is back
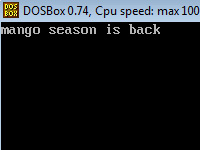
strupr() function in C
Strupr ( ): This function converts the string into upper case.
Syntax
strupr (Name_of_string);
C program to convert a string into uppercase.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”delhi”;
printf (“%s\n”,strupr(str));
return 0;
}
Output
DELHI
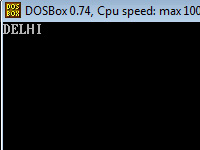
Strrev() function in C
Strrev( ): This function reverses the given string.
Syntax
strrev(Name_of_string);
C program reverse the given string.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”Mumbai”;
printf(“%s\n”,strrev(str));
return 0;
}
Output
iabmuM
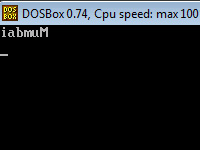
strtok() function in C
strtok ( ): This function breaks the string using a delimiter.
Syntax
strtok(Name_of_string);
C program in which a string is broken into substring.
#include <stdio.h>
#include <string.h>
int main()
{
char str[]=”Hello:World:Hello:Hi:Meet:them”;
char *ptr;
ptr=strtok(str,”:”);
while(ptr!=NULL)
{
printf (“%s\n”,ptr);
ptr=strtok(NULL, “:”);
}
return 0;
}
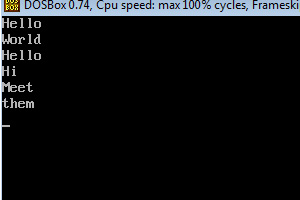
Output
Hello
World
Hello
Hi
Meet
them
Passing strings to function in C
Strings in C is also called the character of the array. Strings pass in a function is similar the way the array is passed.
#include <stdio.h>
//Declaration of print function
void print_string(char str[]);
int main()
{
char str[]=”string”;
print_string(str); //Calling print function
}
//Definition of print function
void print_string(char str[])
{
puts(str);
}
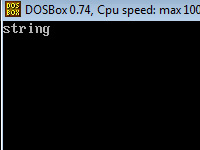
Output
string
Pointers and Character Strings in C
We understand the Pointers and strings with the help of a programming example.
Pointers in C
#include <stdio.h>
int main()
{
char *name=”Shona”;
int length=0;
char *ptr=name;
while (*ptr!=0)
{
ptr++;
length++;
}
printf (“\n length of the string = %d\n”,length);
return 0;
}
Output
length of the string = 5
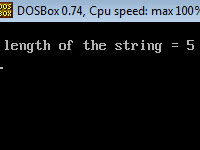