The array is also called a constant pointer. This pointer points to the first element of the array. Whenever you create an array, this pointer is automatically created. So, if you assign an array name to a pointer variable, then there is no need to use an address of the (&) operator before the name of the array. Because as I mentioned earlier an array name is already a constant pointer.
POINTER TO AN ARRAY
#include <stdio.h>
int main ( )
{
// Declaration and initialization of array
int array[5] = {11,22,33,44,55};
int *qtr; int i;
qtr = array;
// Print 0th element of an array
printf (“%d\n”, *qtr);
// Print all elements of an array
for (i=0; i<5; i++)
{
printf (“%d\n”, *(qtr+i));
}
return 0;
}
In C, a pointer to an array is essentially a pointer that holds the address of the first element of the array. This concept can be demonstrated through examples and explanations.
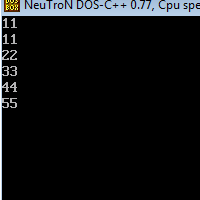
Declaring a Pointer to an Array
When you declare an array, you allocate a block of memory that can hold a specified number of elements. For instance:
int arr[5] = {1, 2, 3, 4, 5};
Here, arr
is an array of 5 integers. You can declare a pointer to this array like this:
int *ptr = arr;
In this case, ptr
is a pointer to an integer, and it points to the first element of arr
.
Accessing Array Elements through a Pointer
Pointer to Array in C Example
You can access the elements of the array through the pointer. For example:
#include <stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
int i;
int *ptr = arr;
for (i = 0; i < 5; i++) {
printf(“%d “, *(ptr + i));
}
return 0;
}
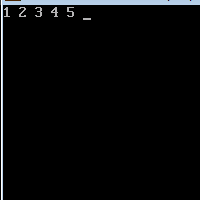
This program will output: 1 2 3 4 5
Pointer to an Array of Fixed Size
Sometimes, you may want to declare a pointer to an array of a specific size. This is useful when dealing with functions that expect an array of a specific length. Here’s an example:
void printArray(int (*ptr)[5]) {
for (int i = 0; i < 5; i++) {
printf(“%d “, (*ptr)[i]);
}
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
printArray(&arr);
return 0;
}
In this example, printArray
it takes a pointer to an array of 5 integers. The &arr
syntax is used to pass the address of the array to the function.
Pointer to an Array vs. Array of Pointers
It’s important to distinguish between a pointer to an array and an array of pointers.
Clarify the difference:
int *arrOfPointers[5]; // Array of 5 pointers to integers
int (*ptrToArray)[5]; // Pointer to an array of 5 integers
- arrOfPointers is an array that can hold 5-pointers to integers.
- ptrToArray is a pointer to an array of 5 integers.
Pointer to 2DArray in C Example
Here’s a more complex example demonstrating the use of pointers to arrays
#include <stdio.h>
int i,j;
void print2DArray(int (*arr)[3], int rows) {
for ( i = 0; i < rows; i++) {
for ( j = 0; j < 3; j++) {
printf(“%d “, arr[i][j]);
}
printf(“\n”);
}
}
int main() {
int arra[2][3] = {{1, 2, 3}, {4, 5, 6}};
print2DArray(arra, 2);
return 0;
}
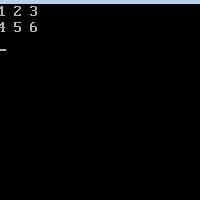
In this example, print2DArray
is a function that takes a pointer to a 2D array with 3 columns. The function prints the contents of the 2D array.