The symbols which are used to perform Arithmetic (mathematical), logical and relational operations in a C language are called C operators.
Types of Operators and Examples.
Operator consists of the following types:
Arithmetic Operators in C:
the addition is denoted by a + sign. We use addition to add two variables.
subtraction is denoted by a – sign
multiplication is denoted by a * sign
the division is denoted by a / sign
modulus (remainder) %: mod operator in a c programming language is used to find the remains(remainder) of some division.
/*Program of Arithmetic Operator*/
#include<stdio.h>
void main()
{
int a,b,c;
clrscr();
printf(“enter the value of a and b and c”);
scanf(“%d%d”,&a,&b);
c=a+b;
printf(“The Answer + is=%d”,c);
c=a-b;
printf(“\nThe Answer -is=%d”,c);
c=a*b;
printf(“\nThe Answer * is=%d”,c);
c=a/b;
printf(“\nThe Answer / is=%d”,c);
c=a%b;
printf(“\nThe Answer % is=%d”,c);
getch();
}
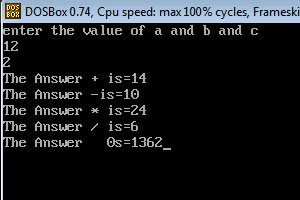
Relational Operators in C:
Relational operators in c language are used to relate one operand with the other. the relational operators are: < Specifies that left-hand operand is less than right-operand
<= Specifies that the left-hand operand is less than or equal to the right-hand operand
> Specifies left-hand operand is greater than the right-hand operand
>= Specifies that the left-hand operand is greater than or equal to the right-hand operand
== Specifies that left-hand operand is equal to the right-hand operand
!= Specifies that the left-hand operand is not equal to the right-hand operand
/*Program of Relational Operator*/
#include<stdio.h>
void main()
{
int x,y;
clrscr();
x=5;
y=3;
printf(“greater=%d”,(x+y)>8);
printf(“\ngreaterequals=%d”,(x+y)>=7);
printf(“\nless than=%d”,(x+y)<9);
printf(“\nless than equals=%d”,(x+y)<=7);
printf(“\ndouble equals=%d”,(x+y)==8);
printf(“\nnot equals=%d”,(x!=y));
getch();
}
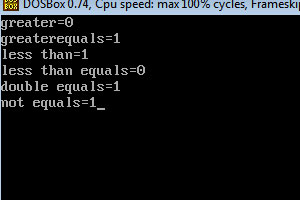
Logical Operators in C:
C programming language provides the following binary logical operators for programs:
&& : Operation AND : returns true if both operands are true
|| : Operation OR : returns true if a single operand or both operands are true
^^ : Operation XOR : returns true if exactly one operand is true
/*Program of Logical Operator*/
#include<stdio.h>
void main()
{
int a,b,c;
clrscr();
a=10,b=20,c=8;
printf(“logical and=%d”,(a<=b)&&(b>c));
printf(“\n logical or=%d”,(b==c)||(b>=a));
printf(“\n logical not=%d”,!(a!=c));
getch();
}
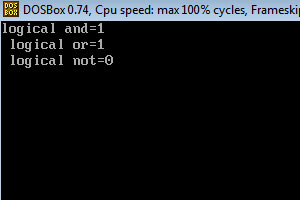
Bitwise Operator in C(binary operator):
C language provides the following binary logical operators for programs:
& : Performs AND operation
| : Performs OR operation
^ : Performs XOR operation
<< : Performs Shift operation i.e. left shifting left-hand operand by the number of bits specified by the right-hand operand
>> : Performs Shift operation i.e. right shifting-left hand operand by the number of bits specified by the right-hand operand.
The shift operators are used to move bits left or right in a given integer value specified. Shifting left will consume empty bit positions on the right-hand side of the result producing zeroes
/*Program of Bitwise Operator*/
#include<stdio.h>
void main()
{
int a,b,c;
clrscr();
a=10,b=11;
c=a&b;
printf(“bitwise and=%d”,c);
c=a|b;
printf(“\nbitwise or=%d”,c);
c=a^b;
printf(“\n bitwise xor=%d”,c);
c=a<<2;
printf(“\n left shift=%d”,c);
c=a>>2;
printf(“\n right shift=%d”,c);
getch();
}
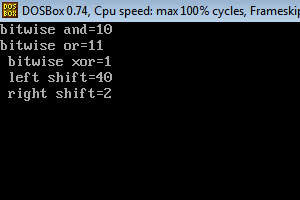
Assignment Operators in C:
C programming language provides the following binary logical operators for programs:
= : Equates left-hand operand equal to the right-hand expression value
+= : Increments the left-hand operand specified by the right-hand expression value
-= : Decrements the left-hand operand specified by the right-hand expression value
*= : Multiply the left-hand operand specified by the right-hand expression value
/= : Divide the left-hand operand specified by the right-hand expression value
%= : Modulo the left-hand operand specified by the right-hand expression value
|= : Performs Bitwise OR operation between the left-hand operand with the right-hand expression value
&= : Performs Bitwise AND operation between the left-hand operand with the right-hand expression value
^= : Performs Bitwise XOR operation between the left-hand operand with the right-hand expression value
/*program of Assignment Operator*/
#include<stdio.h>
void main()
{
int a,b,c;
clrscr();
a=b=c=10;
printf(“a equal=%d”,c);
printf(“\nb equal=%d”,b);
printf(“\n c equal=%d”,a);
a+=2;
printf(“\nplus equal=%d”,a);
a-=2;
printf(“\nminus equal=%d”,a);
a*=2;
printf(“\n multiply equal=%d”,a);
a%=2;
printf(“\n mod equal=%d”,a);
getch();
}
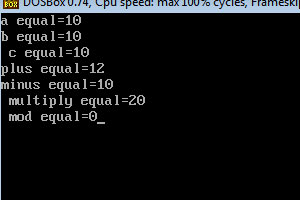