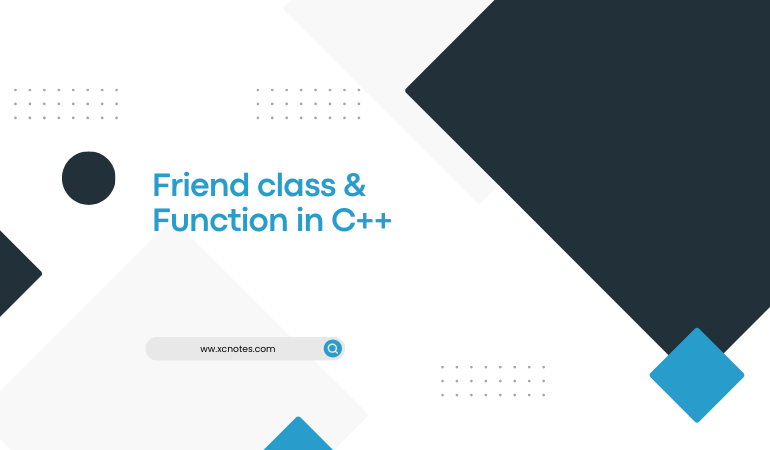
Friend class and Function in C++ with Example
In C++, a friend function is a function that is granted access to the private and protected members of a class. It can be declared inside the class, but it is not a member of that class. A friend function can be useful when you need to allow a function external to the class to access its private or protected members, while still maintaining encapsulation.
To declare a friend function, you need to add a function prototype inside the class declaration, preceded by the friend
keyword.
Here’s an example:
class MyClass {
private:
int privateData;
public:
MyClass(int data) : privateData(data) {}
friend void friendFunction(MyClass& obj);
};
void friendFunction(MyClass& obj) {
// Accessing private member of MyClass
obj.privateData = 42;
}
In the example above, friendFunction
is declared as a friend function inside the MyClass
declaration. It can access the private member privateData
of any MyClass
object.
To declare a friend class, you need to add a friend
keyword followed by the class name inside the class declaration.
Here’s an example:
class MyClass {
private:
int privateData;
public:
MyClass(int data) : privateData(data) {}
friend class FriendClass;
};
class FriendClass {
public:
void modifyData(MyClass& obj, int newData) {
obj.privateData = newData;
}
};
In the above example, FriendClass
is declared as a friend class of MyClass
. It can access the private member privateData
of any MyClass
object through its modifyData
member function.
Both friend functions and friend classes can be helpful in specific situations where you need to allow external functions or classes to access the private or protected members of a class while maintaining encapsulation and data hiding. However, it is generally recommended to minimize the use of friend functions and classes, as they can potentially break encapsulation if used excessively.
In C++ class has a data member and member function. Data members declare private that cannot be accessed outside the class, which means non-member function of the class. But sometimes the situation arises, private data members need to access outside the class. That is done by the friend function. A friend function is called a non-member function, when we create a friend function, we can access private members of the class.
Example
#include<iostream.h>
#include<conio.h>
class xyz
{
private:
int x;
friend void disp();
};
void disp()
{
xyz obj;
obj.x=5;
cout<<“the value of x=”<<obj.x;
}
void main()
{
disp();
getch();
}
Output……
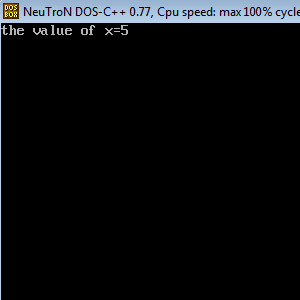
Explanation….
In this example, we create a disp(), which is private. But the function friend means we can access outside the class. The friend function accesses the class variable x by using the dot operator. By using friend functions, the member function of one class can be accessed by another class.