/* Fill up an array,print the array,count number of values,count the number of odd,count the number of even,sum of odd ,sum of even,average,find largest value,find smallest */
#include<iostream.h>
int fill_up(int array[],int size);
void main()
{
int array[10],size;
cout<<“Enter the size of an array:”<<endl;
cin>>size;
fill_up(array,size);
}
int fill_up(int array[],int size)
{
int count=0,even=0,odd=0,even_sum=0,odd_sum=0,largest,smallest;
float avg=0.0,avg_sum=0.0;
int i;
cout<<“Enter the element:”<<endl;
for( i=0;i<size;i++)
{
cin>>array[i];
if(array[i]%2==0)
{
even++;
even_sum+=array[i];
}
else
{
odd++;
odd_sum+=array[i];
}
count++;
avg_sum+=array[i];
}
avg=avg_sum/size;
largest=array[0];
smallest=array[0];
for( i=0;i<size;i++)
{
if(array[i]<smallest)
{
smallest=array[i];
}
}
for( i=0;i<size;i++)
{
if(array[i]>largest)
{
largest=array[i];
}
}
cout<<“The value of the array is:”<<endl;
for( i=0;i<size;i++)
{
cout<<array[i]<<“\t”;
}
cout<<endl;
cout<<“The number of value into the array:”<<count<<endl;
cout<<“The number of even Value is:”<<even<<endl;
cout<<“The number of Odd value is:”<<odd<<endl;
cout<<“The sum of the even number is:”<<even_sum<<endl;
cout<<“The sum of the Odd number is:”<<odd_sum<<endl;
cout<<“The Avarage is:”<<avg<<endl;
cout<<“The Largest value is:”<<largest<<endl;
cout<<“The Lowest value is:”<<smallest<<endl;
return 0;
}
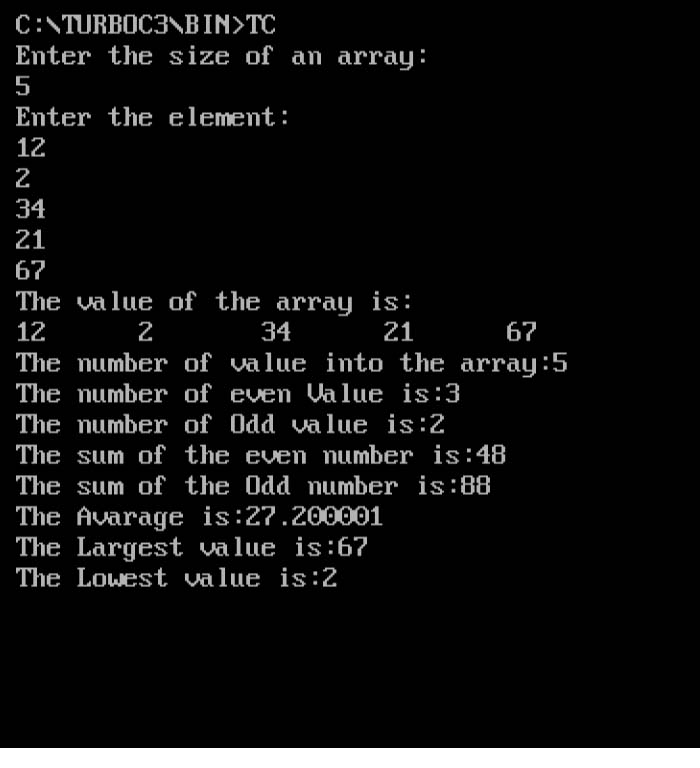