A break statement is one of the branching statements(break, continue) provided by Java, which is also used to control the flow of the program. A break statement is used to exit (stop, terminate)from a running loop program on a condition that is predefined, before the loop completion(condition).
By using break, you can force immediate termination(stop or exit) of a loop, bypassing the conditional expression(for(int aa=0;aa<intarray.length;aa++))and any remaining code in the body of the loop. When a break, the statement is encountered inside a loop(if(intarray[aa]==5)break; ), the loop is terminated and program control resumes at the next statement following the loop.
Break Statement with Example
public class javabreak
{
public static void main(String aba[])
{
int intarray[]=new int[]{1,2,3,4,5,6};//values assign to an array
System.out.println(“Elements less than 5 are”);
for(int aa=0;aa<intarray.length;aa++)
{
if(intarray[aa]==5)
break; //terminate loop if intarray is 5
else
System.out.println(intarray[aa]);
}
}
}

Explanation…
In this example first, we created a class javabreak. In this class, in the main() six elements are signed to an array intarray. After this, the statement elements less than 5 are printed on the screen.
Next, for loop starts with the initialization of the array elements aa, and proceeds with checking the condition<intarray.length. Then, if statement is used to check the condition intarray[aa]==5, if it evaluates to true, the break statement breaks the loop, or else the statement within the else block is executed.
Break Statement with Switch
Break in Switch construct. The break statement in the switch construct is used to terminate the sequential execution of the statement and transfer execution outside the switch block.
When a variable’s value matches the constant specified in the case, the statements within that case block are executed until a break is encountered. If there is no break in the block of that case, the statements of the other case block are also executed until a break is encountered.
Hence, a break acts as a terminator for the execution of statements in a switch-case construct, and then the statements outside the switch-case block are executed.
Switch with break statement
Example….
class switchbreak
{
public static void main(String aa[])
{
char choicee=’y’;
switch(choicee)
{
case ‘y’:
System.out.println(“yes”);
break;
case ‘n’:
System.out.println(“no”);
break;
default:
System.out.println(“pls enter y or n”);
}
}
}
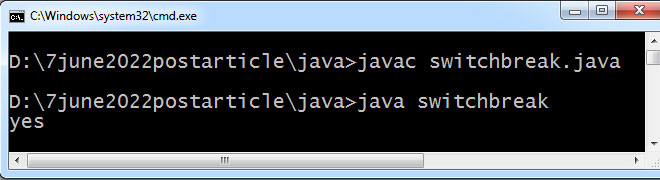
Switch without break statement
class switch_without_break
{
public static void main(String aa[])
{
char choicee=’y’;
switch(choicee)
{
case ‘y’:
System.out.println(“yes”);
case ‘n’:
System.out.println(“no”);
break;
default:
System.out.println(“pls enter y or n”);
}
}
}
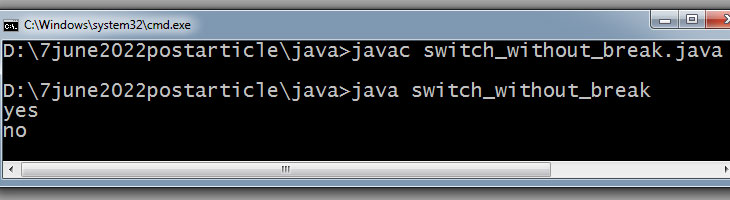
If the break is not written, the control will automatically move to the next case label.
The break statement takes the control out of the switch block after the execution of the case block.