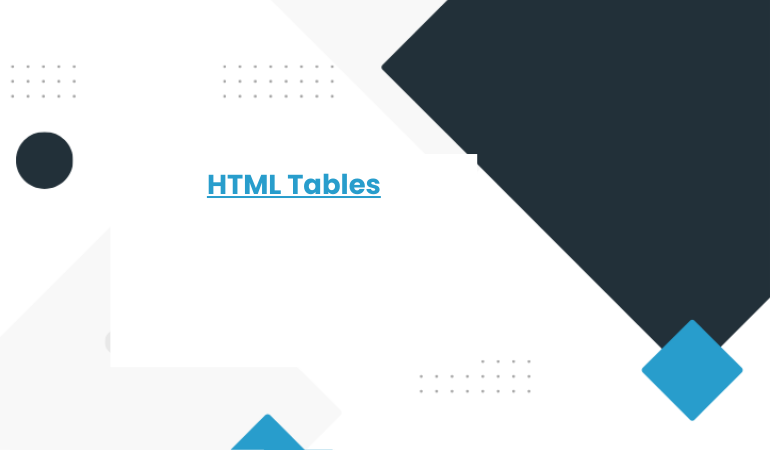
HTML Tables
In HTML, you can create tables using the <table>, <tr>, <td>, and <th> tags. Here’s a basic example of an HTML table(with css):
<!DOCTYPE html>
<html>
<head>
<style>
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 20px;
}
th, td {
border: 1px solid #dddddd;
text-align: left;
padding: 8px;
}
th {
background-color: #ffff00;
}
</style>
</head>
<body>
<h2>Sample Table</h2>
<table>
<thead>
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1, Cell 1</td>
<td>Row 1, Cell 2</td>
<td>Row 1, Cell 3</td>
</tr>
<tr>
<td>Row 2, Cell 1</td>
<td>Row 2, Cell 2</td>
<td>Row 2, Cell 3</td>
</tr>
<tr>
<td>Row 3, Cell 1</td>
<td>Row 3, Cell 2</td>
<td>Row 3, Cell 3</td>
</tr>
</tbody>
</table>
</body>
</html>
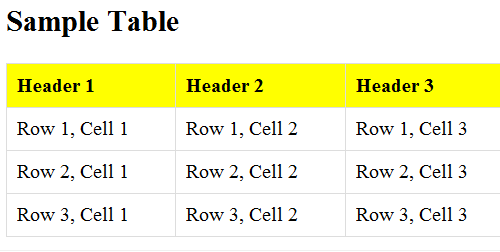
Let’s break down the code:
The <table> element is used to create the table.
Inside the table, the <thead> element is used for the table header (containing column headings), and the <tbody> element is used for the table body (containing the actual data).
Each row in the table is represented by the <tr> (table row) tag.
Within each row, data cells are represented by the <td> (table data) tag for regular cells and <th> (table header) tag for header cells.
CSS styles are applied for basic formatting, such as borders and padding.
Feel free to customize this example based on your specific needs. You can add or remove rows, columns, and styling as required.
In HTML, you can use various attributes to control the structure and appearance of tables. Here are some common attributes used with the <table>, <tr>, <th>, and <td> tags:
<table> Attributes:
border:
Specifies the width of the border around the table and its cells(border=1).
<table border=”1″>
width:
Specifies the width of the table(width is the attribute).
<table width=”100%”>
cellspacing:
Specifies the space between cells.
<table cellspacing=”5″>
cellpadding:
Specifies the space between the cell content and cell border.
<table cellpadding=”10″>
summary:
Provides a summary or description of the table’s content (for accessibility purposes).
<table summary=”This is a summary of the table”>
<tr>, <th>, and <td> Attributes:
align:
Specifies the horizontal alignment of the content within a cell or header.
<th align=”left”>Header</th>
valign:
Specifies the vertical alignment of the content within a cell or header.
<td valign=”top”>Cell Content</td>
colspan:
Specifies the number of columns a cell or header should span.
<td colspan=”2″>Spanning Two Columns</td>
rowspan:
Specifies the number of rows a cell or header should span.
<td rowspan=”2″>Spanning Two Rows</td>
bgcolor:
Specifies the background color of a cell or header.
<th bgcolor=”#e0e0e0″>Header</th>
These attributes provide a range of options for customizing the appearance and behavior of HTML tables. It’s important to note that some attributes, like border, cellspacing, and cellpadding, are considered somewhat outdated, and using CSS for styling is often preferred in modern web development.
HTML Table Example
<html>
<head>
<title></title>
</head>
<body><center><h1 align=left> Table</h1></center>
<table border=”1″ bgcolor=”blue”>
<tr>
<td rowspan=”2″>1,1</td>
<td>1,2</td>
<td>1,3</td>
<td>1,4</td>
</tr>
<tr>
<td>2,1</td>
<td colspan=”2″>2,2</td>
<td>2,3</td>
<td>2,4</td>
</tr>
<tr>
<td>3,1</td>
<td>3,2</td>
<td>3,3</td>
<td>3,4</td>
</tr>
</body>
</html>
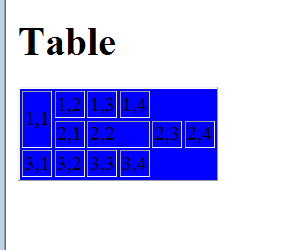