Reversing a Linked list is a useful operation in which we change next to prev, prev to current, and current to next.
/* Program of reverse linked list*/
# include <stdio.h>
# include <malloc.h>
struct nod
{
int info;
struct nod *liink;
}*strt;
main()
{
int i,n,item;
strt=NULL;
printf(“How many nods you want : “);
scanf(“%d”,&n);
for(i=0;i<n;i++)
{
printf(“Enter the item %d : “,i+1);
scanf(“%d”,&item);
create_list(item);
}
printf(” The final liinked list is :\n”);
display();
reverse();
printf(“liinked list after reversing is :\n”);
display();
}/*End of main()*/
create_list(int num)
{
struct nod *q,*tmp;
tmp= malloc(sizeof(struct nod));
tmp->info=num;
tmp->liink=NULL;
if(strt==NULL)
strt=tmp;
else
{
q=strt;
while(q->liink!=NULL)
q=q->liink;
q->liink=tmp;
}
}/*End of create_list() */
display()
{
struct nod *q;
if(strt == NULL)
{
printf(“Oh!List is empty\n”);
return;
}
q=strt;
while(q!=NULL)
{
printf(“%d “, q->info);
q=q->liink;
}
printf(“\n”);
}/*End of display()*/
reverse()
{
struct nod *p1,*p2,*p3;
if(strt->liink==NULL) /*only 1 element*/
return;
p1=strt;
p2=p1->liink;
p3=p2->liink;
p1->liink=NULL;
p2->liink=p1;
while(p3!=NULL)
{
p1=p2;
p2=p3;
p3=p3->liink;
p2->liink=p1;
}
strt=p2;
}/*End of reverse() */
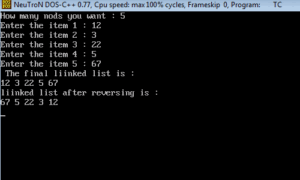