What is a variable?
Variable is the name of the memory location from which we store the value. This is clear in the above example. A name that holds value. We can use variables to represent data.
Representing attributes by variables. An attribute of an object can be referred to by a variable name that can store a value. E.g. age of a student or person can be represented as an integer number(int age=45). Hence declared as int age; the value may also be initialized there and then with the declaration.e.g int age=12;
Types of Variables in Java:
Numeric Types:
Integer type:holds all whole numbers(+ve,-ve)
e.g. int a;
Float type: stores all real numbers(containing decimal points)
e.g. float b;
Non-Numeric Types:
Character Type:holds only 1 character(alphabet,digit or special character)
e.g. char c;
String Type:holds more than one character(words,sentence,paragraph)
e.g. String s;
Initializing a Variable
Datatype declaration default initialization
Integer int a; a=0;
Float float b; b=0.0;
Double double c; c=0.0;
Character char d; d= ‘ ’;
String String e; e=” “;
Boolean Boolean f; f=false;
e.g.
int a=67; //67 is stored in the variable a which is an integer type
float b=2.45; //2.45 is stored in the variable b which is a float type
char c=’r’; //r character is stored in the variable which is a character type
RULES FOR NAMING VARIABLES:
- May have any no. of characters.
- May contain alphabet(w,r),digit(4,5) and underscore(_);
- Underscore can be applied in btw the characters(like first_name);
- The name should be meaningful(int age, float salary)
Variable to be Invalid
- The name should not start with a digit(like int 9a), or a special character.
- The name should not include a space(like String first name)
class variableexample
{
public static void main(String aa[])
{
int a; //variable declaration
a=9; //constant value
if(a>0) //means if a is greater than 0 then message print positive ortherwise negative.
{
System. out. print(“positive”);//message print if condition is true
}
else
{
System. out. print(“negative”);//message print if condition is false
}
}
}
Save this program……. Variableexample.java
Run……javac variableexample.java
Java variableexample
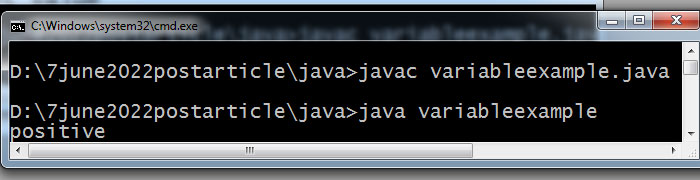
In this example, a is the variable of integer type means it can store 2 bytes of data because integer ( int )Data type can store 2bytes. The data type defines the size of the variable. The size of the variable depends upon the data type for example we use the “char” data type then it can use 1byte of data so this is clear that every variable depends upon data type . Without datatype, the variable is nothing in any programming.