Factorial number definition:
Factorial of n number is the product(*) of all positive integer(1*2*3*4*5)
Denoted :
5! Means 5*4*3*3*1=120 here 51 means 5 factorial
3! Means 3*2*1=6 here 3! Means 3 factorial
It is very interesting to note that the program with one type of loop can be converted to another program
While, a do-while, for loop to produce the same result.
To find the factorial of a given number with for loop
class factloop1
{
public static void main(String aa[])
{
int a;
int n=10;
double fact;
fact=1;
for(a=1;a<=n;a++)
{
fact=fact*a;
}
System.out.println(“factorial no=”+fact);
}}

To find the factorial of a given number with a do-while loop
class factloop2
{
public static void main(String aa[])
{
int a;
int n=10;
double fact;
fact=1;
a=1;
do
{
fact=fact*a;
a++;
}
while(a<=n);
System.out.println(“factorial=”+fact);
}
}
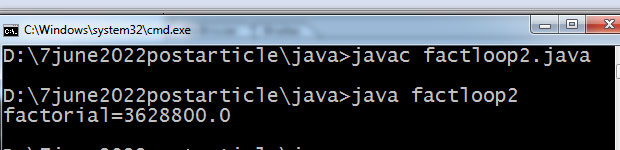
Factorial of a number with a while loop
class factloop3
{
public static void main(String aa[])
{
int a;
int n=10;
double fact;
fact=1;
a=1;
while(a<=n)
{
fact=fact*a;
a++;
}
System.out.println(“factorial=”+fact);
}
}
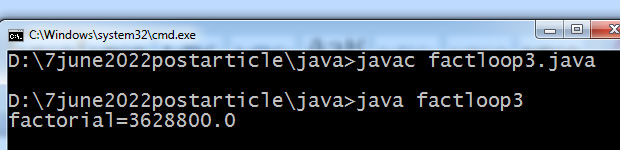
In the above examples, we use different loops like while,do-while, for loop
For loop is used to repeat one or more than one statement a specified number of times.
The while evaluates test expression before entering further into the loop
The do-while loop is different from the while loop in this the loop is evaluated first and thereafter evaluates the test expression.
Factorial in Java using Scanner
import java.util.Scanner; //this package is used for scanner function
class Factorialscanner
{
public static void main(String arxc[])
{
int n, c, fact = 1;
System.out.println(“Enter an integer to calculate it’s factorial”);
Scanner in = new Scanner(System.in);
n = in.nextInt();
if ( n < 0 )
System.out.println(“Number should be non-negative.”);
else
{
for ( c = 1 ; c <= n ; c++ )
fact = fact*c;
System.out.println(“Factorial of “+n+” is = “+fact);
}
}
}
